2017-03-10-数组冒泡排序
实例1:
给定一个数组:6,1,7,5,8,2,3,9,4,0找出最大数组中的最大值
思路:先将数组进行排序,然后直接输出最大值
核心要点:冒泡排序算法
给出一个数组,数组中的第一个数依次和后面的每个数比较,如果后面的数比前面的数小,就将两个数交换位置,直到第一个数和最后一个数比较完,
然后第二个数再依次和后面的每个数比较,如果后面的数比它小,则交换位置。直到数组中的倒数第二个数和最后一个数比较完毕。则整个数组就进行了一次冒泡排序。
如果数组长度为n,则进行了n/2次比较
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public void test2(){ int[] a={6,1,7,5,8,2,3,9,4,0}; for(int i=0;i<a.length;i++){ for(int j=i+1;j<a.length;j++){ if(a[i]>a[j]){ a[i]=a[i]+a[j]; a[j]=a[i]-a[j]; a[i]=a[i]-a[j]; } } } System.out.println("给定数组的最大值为:"+a[a.length-1]); }
|
实例2:
找出给定数组(4,2,20,7,1,9,3)的最大值(不用排序)
思路:设定一个最大值,然后将假设的最大值和数组中的每个数比较,如果假设的最大值比数组中的某个数小,则将数组中的这个数赋给这个最大值。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
|
public void test4(){ int[] a={4,2,20,7,1,9,3}; int max=a[0]; for(int i=1;i<a.length;i++){ if(max<a[i]){ max=a[i]; } } System.out.println("数组中最大的数为:"+max); }
|
实例3:
找出给定数组{4,2,20,7,1,9,3}中的元素的最大值和最小值,然后将他们互换
思路:先将数组中的最大值和最小值找出来同时记录最大值的下标和最小值的下标,最后把最大值赋给数组中的最小值,最小值赋给数组中的最大值
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
public void test3(){ int[] a={4,2,20,7,1,9,3}; int max=a[0]; int maxLocation=0; int min=a[0]; int minLocation=0; for(int i=1;i<a.length;i++ ){ if(max<a[i]){ max=a[i]; maxLocation=i; } if(min>a[i]){ min=a[i]; minLocation=i; } } a[maxLocation]=min; a[minLocation]=max; System.out.println("交换之后的数组"); for(int i=0;i<a.length;i++){ System.out.print(a[i]+" "); } }
|
实例4:
将给定数组(4,2,20,7,1,9,3)从大到小排序
思路:冒泡排序
1.我自己的冒泡思路
2.正宗的冒泡思路
我自己的冒泡思路核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
public void test5(){ int[] a={4,2,20,7,1,9,3}; System.out.println("排列前的数组:"); for(int i=0;i<a.length;i++){ System.out.print(a[i]+" "); } System.out.println(); for(int i=0;i<a.length;i++){ for(int j=i+1;j<a.length;j++){ if(a[i]<a[j]){ a[i]=a[i]+a[j]; a[j]=a[i]-a[j]; a[i]=a[i]-a[j]; } } } System.out.println("排列后的数组:"); for(int i=0;i<a.length;i++){ System.out.print(a[i]+" "); } System.out.println(); }
|
正宗的冒泡思路核心代码:
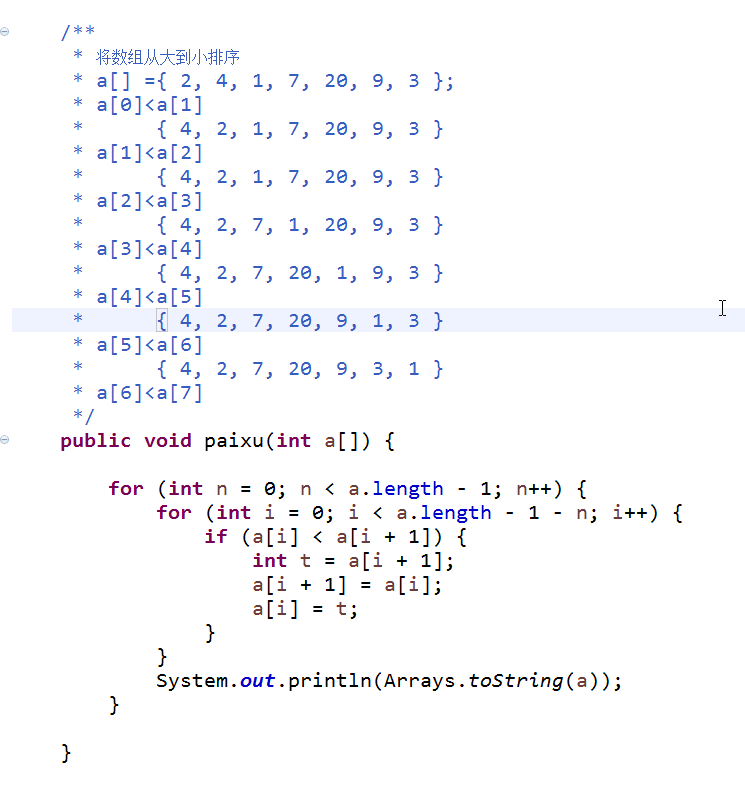
正宗的冒泡思路:(从大到小排列:将小数往下冒)
第一个数和第二个数比较,然后将小的一个数往后面挪,知道挪到最后一位,就得到了第一个最小的数了。将小数往下冒。
关键的是想清楚两次循环的次数,还有中间重复的次数。
我的冒泡思路:(从大到小排列:将大数往上冒)
第一个数依次和后面的每个数比较,然后将大的数放在第一个数的位置。直接将大数冒上来。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
|
public void test7() { int[] a = { 5, 7, 2, 9, 1, 8, 3, 2, 0, 6, 4 }; for (int n = 0; n < a.length - 1; n++) { for (int i = 0; i < a.length - 1 - n; i++) { if (a[i] < a[i - 1]) { a[i] = a[i] + a[i - 1]; a[i - 1] = a[i] - a[i - 1]; a[i] = a[i] - a[i - 1]; } } } }
|
实例5:
制作一个猜数游戏,写一个类Guess,
这个类有一个int型成员变量n。
n里面存储的数必须是一个4位整数,
且这个整数的每一位上的数都不同。
实例化Guess类对象A,B,利用随机数给A对象的n赋值,比较A.n和B.n,
如果完全相等输出“完全正确”,否则输出”A(a)B(b)”,
a表示数字正确且数字位置正确的个数,
b表示数字正确的个数。
创建一个变量用来记录B从开始回答到完全答对的次数。
思路:将n的个十百千位的数据都设置为类的属性,在构造方法里面限定四位数,只要限制随机数在1000~10000之间就行了,然后把随机生成的数的个十百千位都拆分出来,然后再判断每个位置的数都不相同就行了。接下来的难点就是在生成A(a)B(b)这里,只要逻辑思维正确就可以了,就是位置相同,大小相同很有趣。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81
| public class Demo { public int n; int single; int tens; int hundreds; int thousands; public Demo(){ while(true){ n=(int)(Math.random()*9000+1000); single=n%10; tens=n%100/10; hundreds=n%1000/100; thousands=n/1000; if(single!=tens&&single!=hundreds&&single!=thousands&&tens!=hundreds&&tens!=thousands&&hundreds!=thousands){ break; } } } public static void main(String[] args) { Demo d1=new Demo(); d1.test(d1); } public void test(Demo d1) { int count=0; while(true){ int a=0; int b=0; Demo d2=new Demo(); System.out.print(d1.n+" "); System.out.println(d2.n); count++; if(d1.n==d2.n){ System.out.println("完全正确。共猜了"+count+"次"); break; }else{ if(d1.single==d2.single){ a++; } if(d1.tens==d2.tens){ a++; } if(d1.hundreds==d2.hundreds){ a++; } if(d1.thousands==d2.thousands){ a++; } if(d1.single==d2.single||d1.single==d2.tens||d1.single==d2.hundreds||d1.single==d2.thousands){ b++; } if(d1.tens==d2.single||d1.tens==d2.tens||d1.tens==d2.hundreds||d1.tens==d2.thousands){ b++; } if(d1.hundreds==d2.single||d1.hundreds==d2.tens||d1.hundreds==d2.hundreds||d1.hundreds==d2.thousands){ b++; } if(d1.thousands==d2.single||d1.thousands==d2.tens||d1.thousands==d2.hundreds||d1.thousands==d2.thousands){ b++; } System.out.println("A("+a+")B("+b+")"); } } }
}
|
实例6:
* 现在有三个类:Tv代表电视类,Family代表家庭类、Main作为程序入口
*
* Tv里有一个int类型的变量channel,代表电视频道,可以通过两个方法setChannel(int i)、getChannel()设置
* 和得到当前的电视频道,有一个Tv类型得到变量t,可以通过buyTv(Tv tv)方法给t初始化,通过remoteContorl(int m)方法换台,
* 还有一个seeTv()方法看电视
*
*
* 显示如下:
* Tv的频道是:5
* 唐鑫开始看电视节目:体育频道
* 唐鑫将电视更换到2频道
* TV的频道是:2
* 唐鑫开始看电视节目:经济频道
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122
| package com.share.demo03_11;
public class Television { private int channel;
public Television(){ channel=5; } public int getChannel() { return channel; }
public void setChannel(int channel) { this.channel = channel; } public String showProgram(){ String program=""; switch (channel) { case 1: program="综合频道"; break; case 2: program="经济频道"; break; case 3: program="综艺频道"; break; case 4: program="中文国际频道"; break; case 5: program="体育频道"; break; case 6: program="电影频道"; break; case 7: program="农业军事频道"; break; case 8: program="电视剧频道"; break; case 9: program="纪录频道"; break; case 10: program="科教频道"; break; case 11: program="戏剧频道"; break; case 12: program="法制频道"; break; case 13: program="新闻频道"; break; case 14: program="少儿频道"; break;
default: program="综合频道"; break; } return program; } }
public class Family { public String name; public Television t; public Family(){ Scanner sc=new Scanner(System.in); System.out.print("请输入您的名字:"); name=sc.next(); } public void buyTelevision(Television tv) { t=tv; }
public void remoteControl(int in) { System.out.println(name+"将电视更换到:"+in+"频道"); t.setChannel(in); }
public void seeTelevision() { System.out.println("TV的频道是:"+t.getChannel()); System.out.println(name+"开始看电视节目:"+t.showProgram()); } }
public class Main { public static void main(String[] args) { Main m=new Main(); m.show(); } public void show(){ Television t=new Television(); Family f=new Family(); f.buyTelevision(t); f.seeTelevision(); Scanner sc=new Scanner(System.in); System.out.println("请输入您想要观看的频道数:(仅限数字1~14)"); int in=sc.nextInt(); f.remoteControl(in); f.seeTelevision(); } }
|