2017-03-27-内部类,随机不重复原理
一周总结:
不太重视理论知识的整理,过于在意算法和逻辑,导致理论过于散乱。
三个理论学习方法论体系:
1.有些不用记得知识,只记住最重要的就行了
2.把知识的大纲理清楚,但是不用记住
3.感觉很重要,弄清楚来龙去脉
实例1:
生成一个0~9间的随机不重复数组
思路:
随机不重复原理:
重要思路,通过设置标志判断重复,达到不重复。
判断重复,直接通过改变多个元素为一个标志,则能够简单实现重复判断。
这个思路其实在前面做棋盘中的随机位置的时候就已经有过相似的思路,只是自己不会 触类旁通 举一反三。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| package com.share.demo03_27;
import java.util.Arrays;
public class Test { public static void main(String[] args) { test(); }
public static void test() { int[] a = new int[10]; int[] b = new int[10]; for (int i = 0; i < 10; i++) { a[i] = i; } for (int i = 0; i < 10; i++) { int r = 0; do { r = (int) (Math.random() * 10); } while (a[r] == -1);
b[i] = a[r]; a[r] = -1; } System.out.println(Arrays.toString(b)); } }
|
实例2:
已知有十六支男子足球队参加比赛,写一个程序,把这16球队随机分配4个组。(可以使用随机数,也可以不使用随机数)
参加比赛的球队以国家命名,以下是参数国家:
科特迪瓦,阿根廷,澳大利亚,塞尔维亚,荷兰,尼日利亚,日本,美国,中国,新西兰,巴西,比利时,韩国,喀麦隆,洪都拉斯,意大利
思路:
1.随机不重复原理,使用数组;
2.随机不重复原理,使用集合List;
3.使用集合Set。
核心代码:
思路1:
使用数组核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public static void test6(){ String[] a = { "科瓦迪亚", "阿根廷", "澳大利亚", "塞尔维亚", "荷兰", "尼日利亚", "日本", "美国", "中国", "新西兰", "巴西", "比利时", "韩国", "喀麦隆", "洪都拉斯", "意大利" }; String[][] s=new String[4][4]; for(int i=0;i<s.length;i++){ for(int j=0;j<s[i].length;j++){ int r=0; do{ r=(int)(Math.random()*16); }while(a[r].equals("flag")); s[i][j]=a[r]; a[r]="flag";
} } for(String[] s1:s){ System.out.println(Arrays.toString(s1)); } }
|
图文详解:
思路2:
使用list核心代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| public static void test() { String[] a = { "科瓦迪亚", "阿根廷", "澳大利亚", "塞尔维亚", "荷兰", "尼日利亚", "日本", "美国", "中国", "新西兰", "巴西", "比利时", "韩国", "喀麦隆", "洪都拉斯", "意大利" };
String[][] a1 = new String[4][4]; int[] a2 = new int[16];
List<Integer> list = new LinkedList<Integer>(); for (int i = 0; i < 16; i++) { list.add(i); } for (int i = 0; i < a2.length; i++) { int r = (int) (Math.random() * 16); while (list.indexOf(r) == -1) { r = (int) (Math.random() * 16); } a2[i] = r; list.remove(list.indexOf(r)); }
int count = 0; for (int i = 0; i < a1.length; i++) { for (int j = 0; j < a1[i].length; j++) { int ran = a2[count]; a1[i][j] = a[ran]; count++; } }
for (String[] s : a1) { System.out.println(Arrays.toString(s)); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public static void test5(){ String[] a = { "科瓦迪亚", "阿根廷", "澳大利亚", "塞尔维亚", "荷兰", "尼日利亚", "日本", "美国", "中国", "新西兰", "巴西", "比利时", "韩国", "喀麦隆", "洪都拉斯", "意大利" }; List<String> list=new ArrayList<String>(); for(String s:a){ list.add(s); } while(list.size()>0){ int index=(int)(Math.random()*list.size()); System.out.println(list.remove(index)); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public static void test5() { String[] a = { "科瓦迪亚", "阿根廷", "澳大利亚", "塞尔维亚", "荷兰", "尼日利亚", "日本", "美国", "中国", "新西兰", "巴西", "比利时", "韩国", "喀麦隆", "洪都拉斯", "意大利" }; List<String> list = new ArrayList<String>();
for (String s : a) { list.add(s); } String[][] s = new String[4][4];
for (int i = 0; i < s.length; i++) { for (int j = 0; j < s[i].length; j++) { if (list.size() > 0) { int index = (int) (Math.random() * list.size()); s[i][j] = list.remove(index); } } } for(String[] s1:s){ System.out.println(Arrays.toString(s1)); }
}
|
思路3:使用set核心代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public static void test1() { String[] a = { "科瓦迪亚", "阿根廷", "澳大利亚", "塞尔维亚", "荷兰", "尼日利亚", "日本", "美国", "中国", "新西兰", "巴西", "比利时", "韩国", "喀麦隆", "洪都拉斯", "意大利" };
Set<String> set = new HashSet<String>(); for (String s : a) { set.add(s); } String[][] array = new String[4][4];
for (int i = 0; i < array.length; i++) { for (int j = 0; j < array[i].length; j++) { Iterator<String> it = set.iterator(); if (it.hasNext()) { array[i][j] = it.next(); set.remove(array[i][j]); } } } for (String[] t : array) { System.out.println(Arrays.toString(t)); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package com.share.test_3_27;
import java.util.ArrayList; import java.util.List;
public class ShangJi4 { public static void main(String[] args) { String s = "科特迪瓦,阿根廷,澳大利亚,塞尔维亚,荷兰,尼日利亚,日本,美国,中国,新西兰,巴西,比利时,韩国,喀麦隆,洪都拉斯,意大利"; String name[] = s.split(","); List<String> list = new ArrayList<String>(); for (String string : name) { list.add(string); } while (list.size() > 0) { int num = (int)(Math.random()*list.size()); if(list.size()==16){ System.out.println("\nA组:"); }else if(list.size()==12){ System.out.println("\nB组:"); }else if(list.size()==8){ System.out.println("\nC组:"); }else if(list.size()==4){ System.out.println("\nD组:"); } System.out.print(list.remove(num) + "\t"); } } }
|
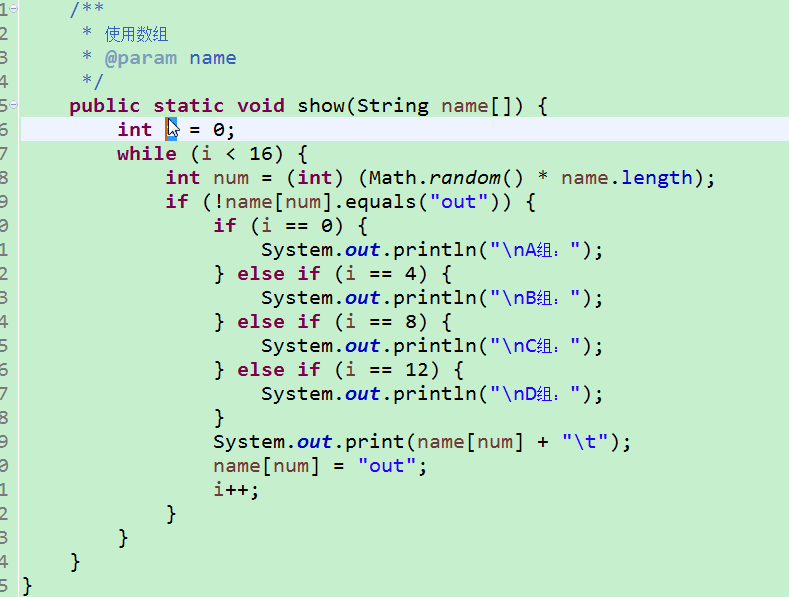
实例3:
1 2 3 4 5 6 7 8 9 10 11 12
| 将二维数组int[][] a={{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15},{16,17,18,19,20}};
显示为下面这种格式:
01 02 03 04 05
10 09 08 07 06
11 12 13 14 15
20 19 18 17 16
|
思路:
将二维数组输出,判断正序 和反序情况,正序输出,反序输出
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| package com.share.test_03;
public class Test03 { public static void main(String[] args) { test(); } public static void test(){ int[][] a={{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15},{16,17,18,19,20}}; for(int i=0;i<a.length;i++){ if(i%2!=0){ for(int j=a[i].length-1;j>=0;j--){ if(a[i][j]<10){ System.out.print("0"); } System.out.print(a[i][j]+" "); } }else{ for(int j=0;j<a[i].length;j++){ if(a[i][j]<10){ System.out.print("0"); } System.out.print(a[i][j]+" "); } } System.out.println(); } } }
|
实例4:
在外部类中创建内部类,内部类中使用外部类同名的属性初始化对象,在外部类中的main方法中创建内部类的对象,并输出显示内部类的成员。
思路:
主要是用到内部类的语法
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| package com.share.demo03_27;
public class OuterClass { public String name; public OuterClass(){ } public OuterClass(String name){ this.name=name; } public class InnerClass { private String name; int age; public char sex;
public InnerClass(){ this.name=OuterClass.this.name; } public InnerClass(String name){ this.name=OuterClass.this.name; } public String getName() { return name; }
public void setName(String name) { this.name = name; } public void showInner(){ System.out.println(""+name); } } public static void main(String[] args) { OuterClass out=new OuterClass("内部类的初始值"); OuterClass.InnerClass in=out.new InnerClass(); in.showInner(); } }
|