2017-03-28-内部类-IO流
总结昨天:
效率太低。
学习模型:
1.对于松散繁杂的简单知识只用将容易记错的知识特殊标记重点记忆和理解就行了
2.对于一整块体系的知识,需要将体系框架的思路理清楚,从每个概念的产生的原因开始到高级应用思路提纲挈领
3.对于总体知识体系,只要了解框架就行了
4.对于一些和其他知识关系不大的内容整理出专题特殊归类就行了
5.总结理论和实践中的问题,把理论和实践中的规律专题化。
聚散结合
细碎简答的知识就用散的方法,不用浪费精力把所有的知识都记住,只要记住某些易错点之类的重点就行了。
系统复杂的知识就用聚的方法,把思路理清楚,通过条理将复杂的知识系统化,只要记住知识纲领和框架就行了。
某些系统中的重点知识,但是和系统中的联系不是很大,也可以使用散的方式来记忆,将其独立出来重点记忆,将其特殊化。
具体学习java的过程中需要做得事:
1.将面向对象之前各种细碎的知识中找到重点,然后特殊重点记忆
2.将面向对象和后面的知识都系统化,归纳出理论的框架,找出框架内部知识之间的相互关系,弄清楚为什么会产生这个理论的概念。
通过逻辑推导就可以记住最根本的道理,后面的情况自然就顺通了。
3.每个知识点与对应的代码实现都要非常熟悉,单方面了解知识点或者熟悉代码都是不行的,必须理论与实践结合。
4.实例的整理,50道算法题,语法和算法都要兼顾。
归纳学习模型:
分为两条线:
1、语法 主要是java中的理论
2、算法 实际问题中的逻辑
将这两条线可以推广到立刻学习中的理论与问题,理想与现实的关系。
知识点0:
匿名内部类可以是实现接口的类,也可以是对类继承的类,可以定义父类中没有的其他方法,但是访问不到。
知识点1:
hashSet中存储数字数据无法识别hashCode的值,无法实现无序,将整型数据转换成字符串就能够实现无序。
如果数据量太小,一般会和存进来的顺序相同。
演示代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.share.demo03_28;
import java.util.HashSet; import java.util.Set;
public class Test { public static void main(String[] args) { test(); } public static void test(){ Set<String> set=new HashSet<String>(); for(int i=0;i<100;i++){ set.add(String.valueOf(i)); } System.out.println(set); } }
|
图文详解:
其他:
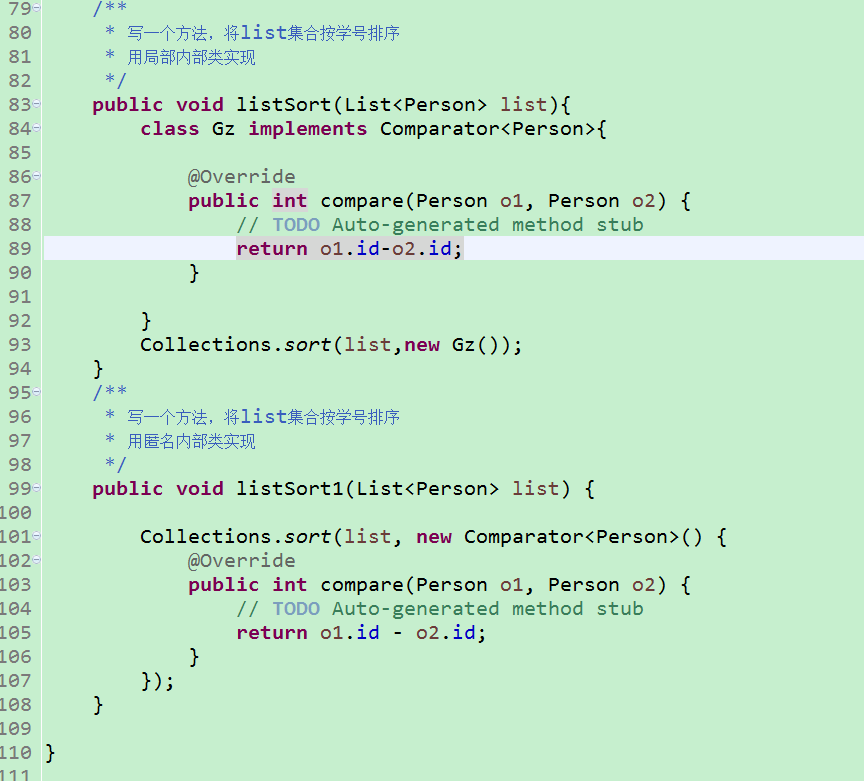
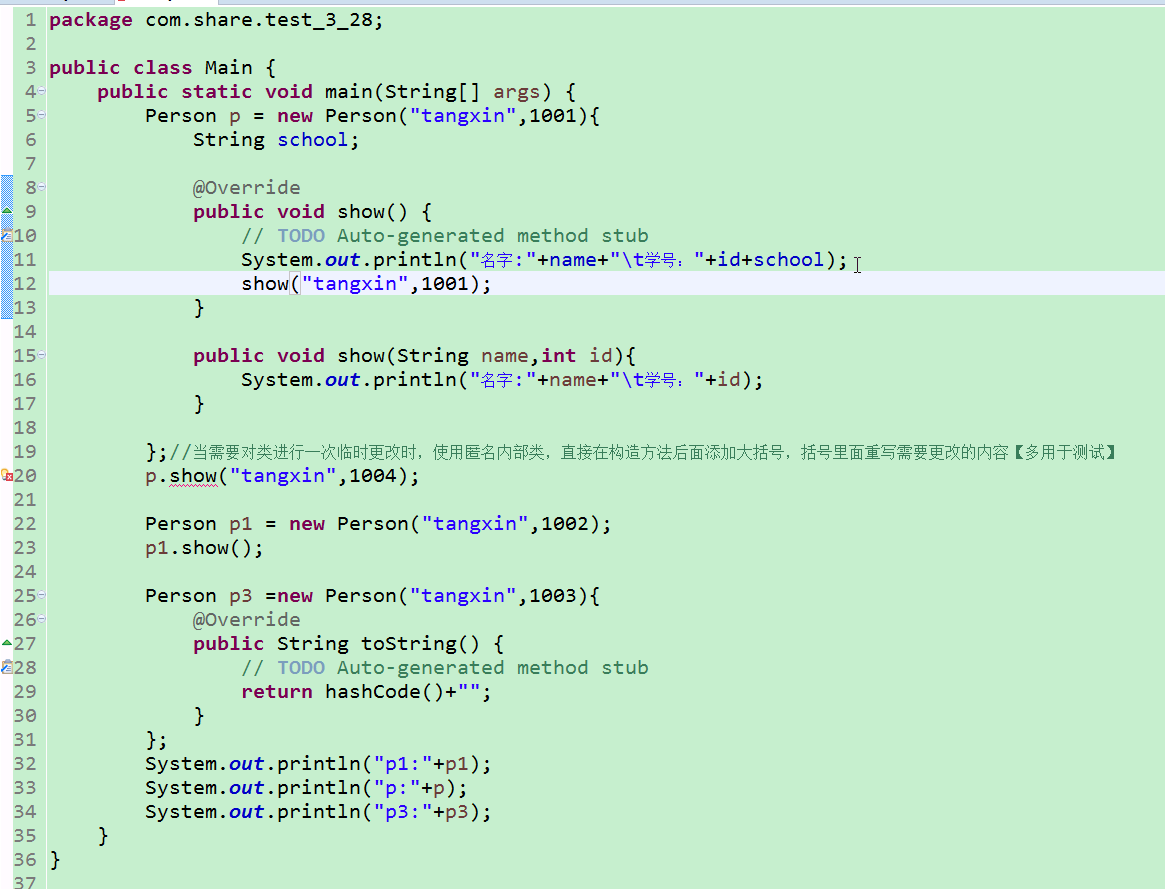
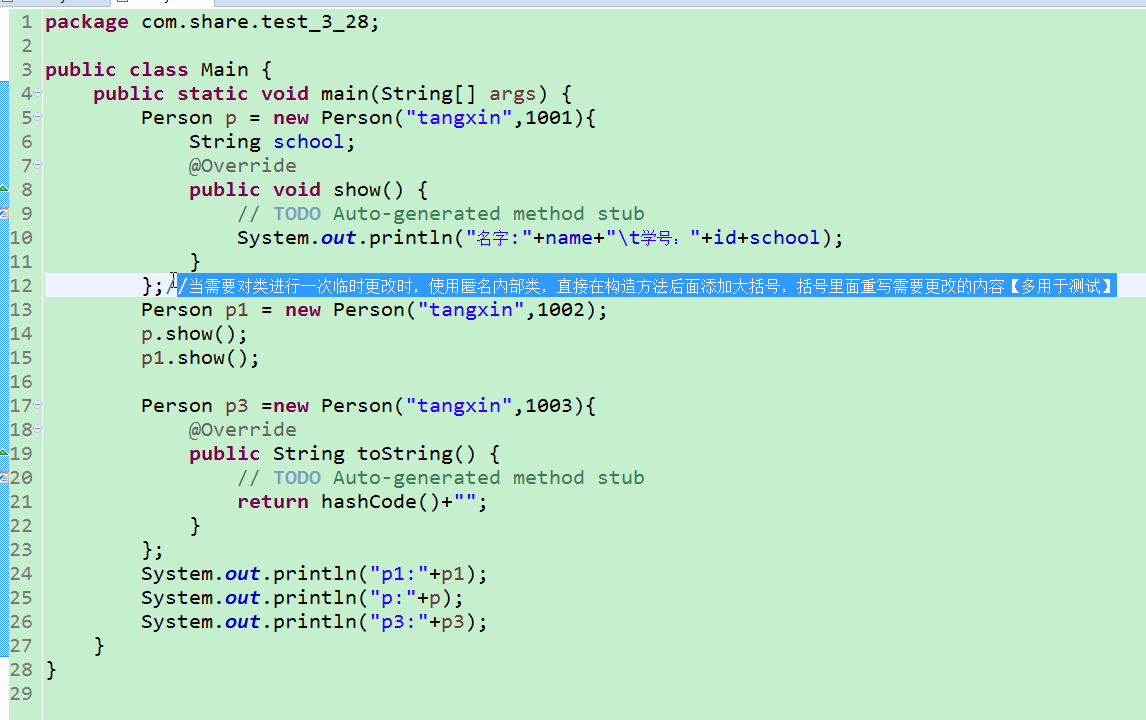
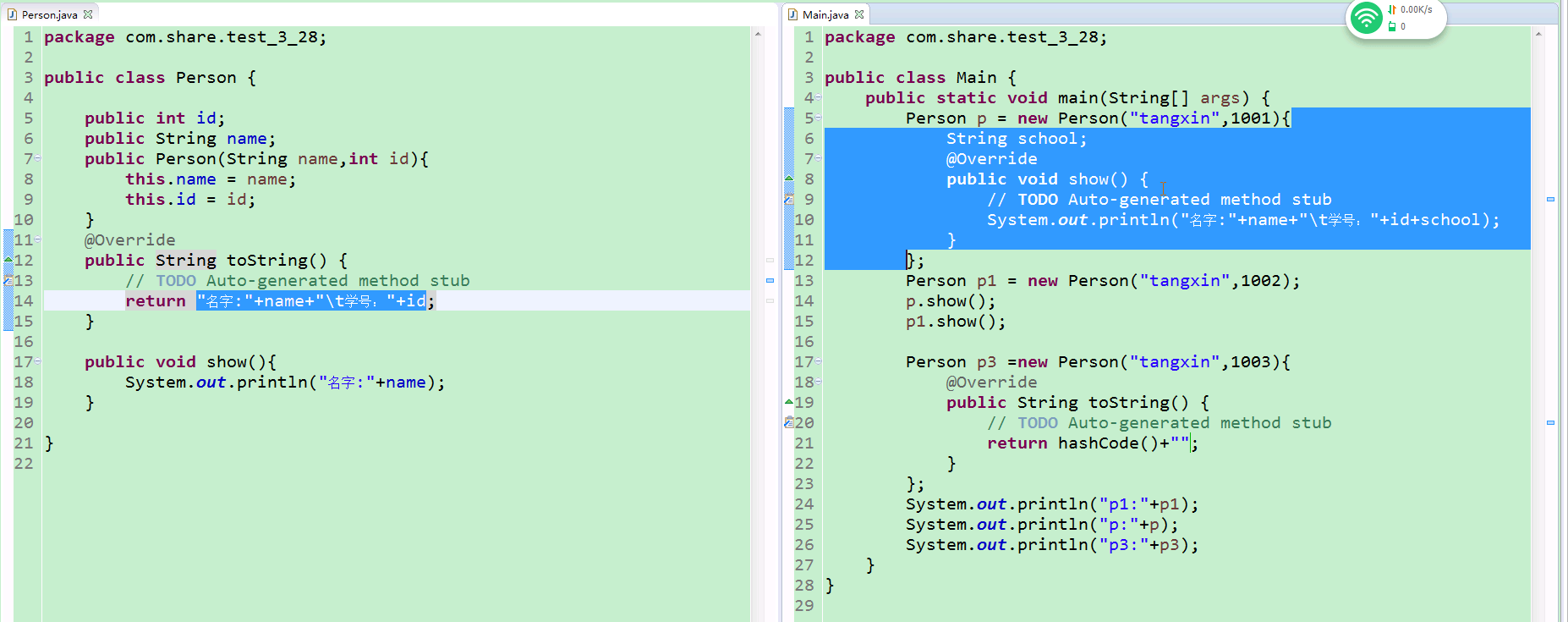
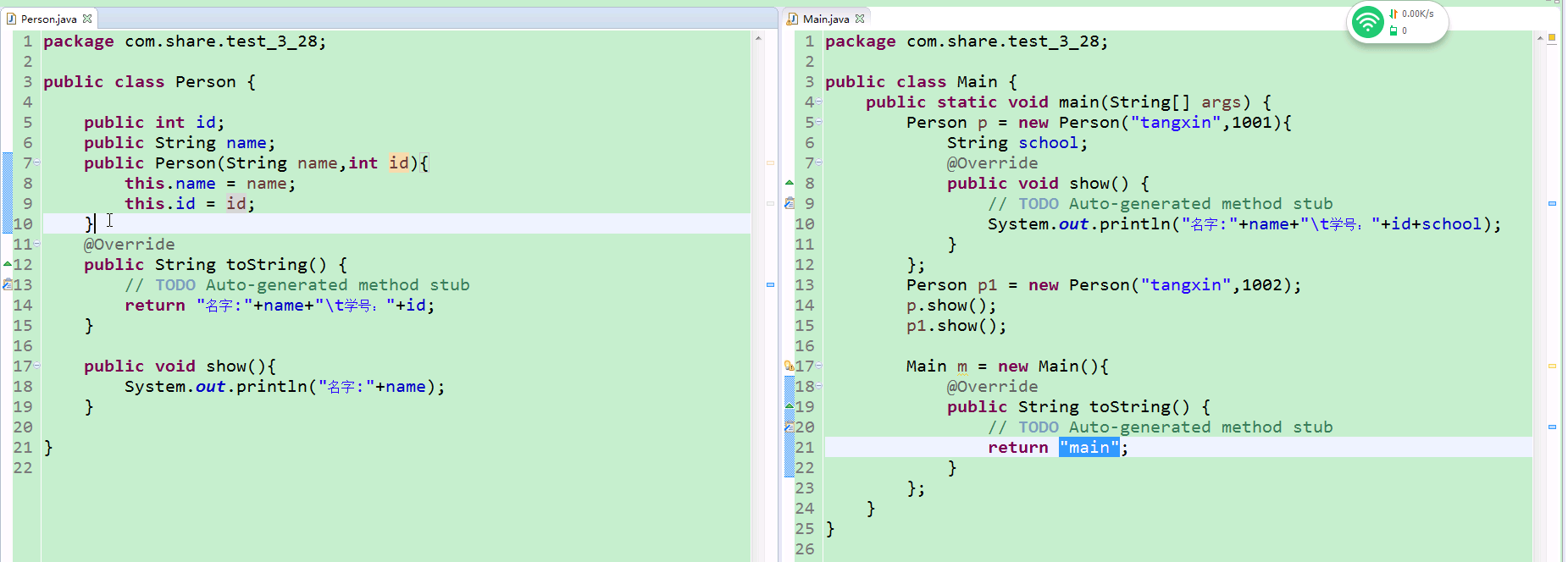
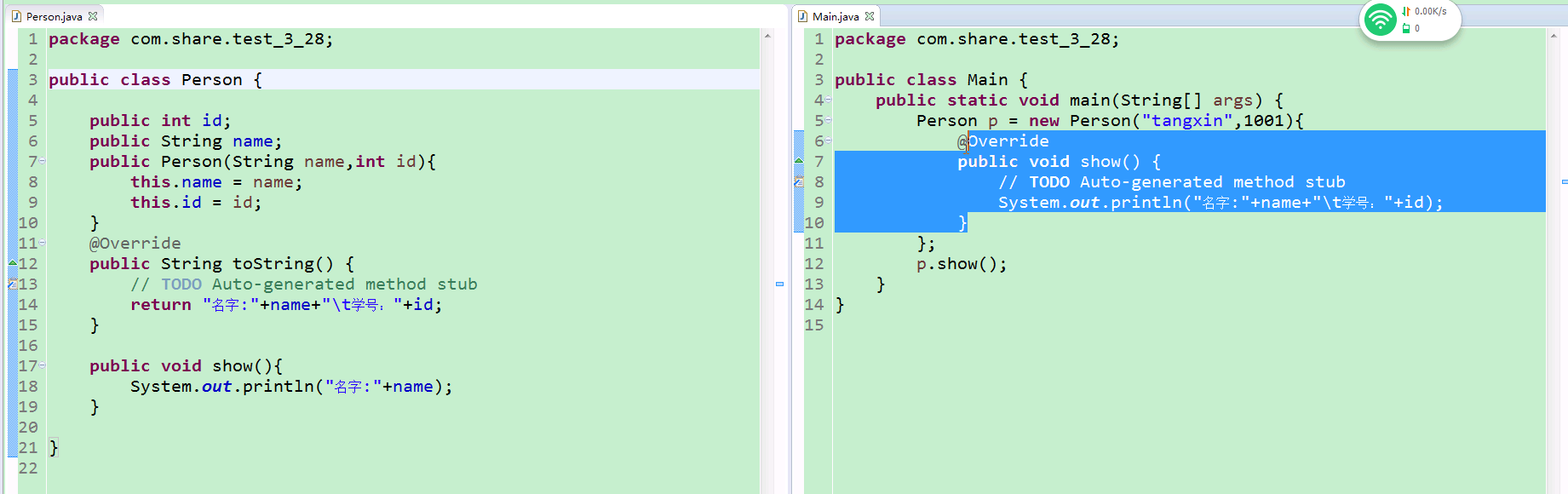
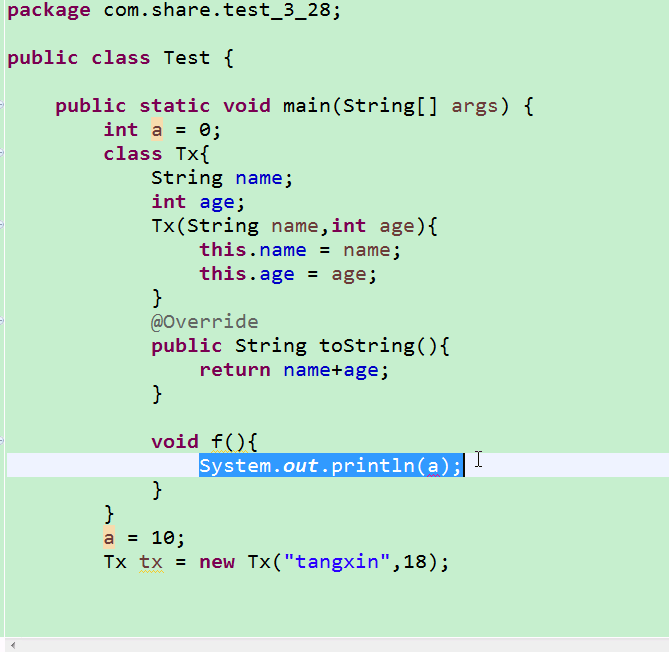
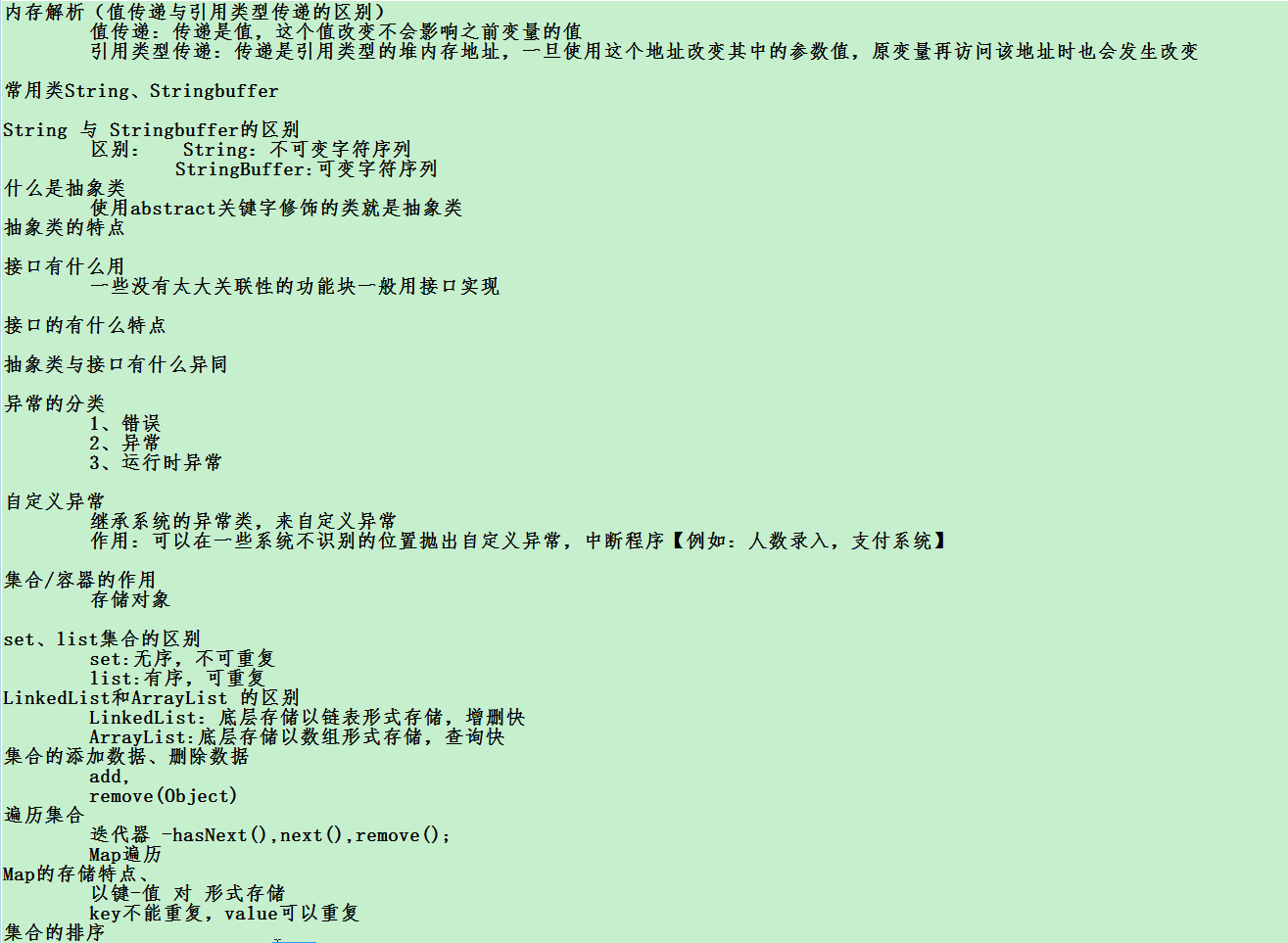
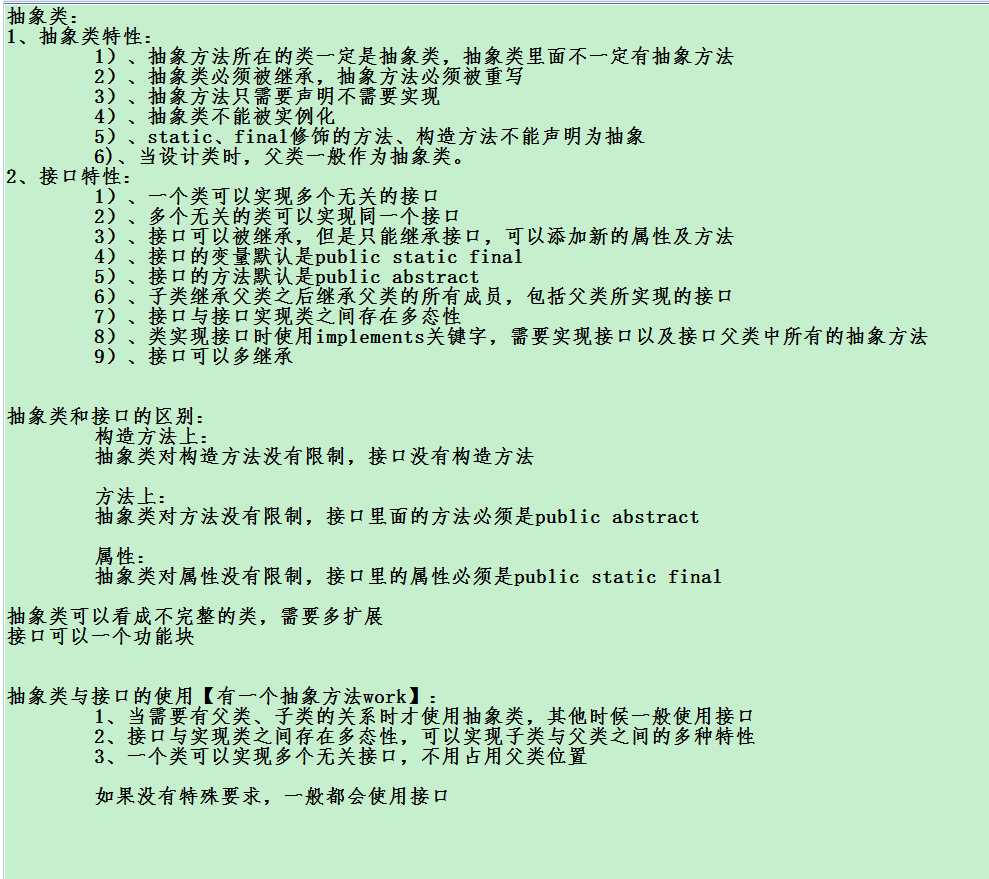
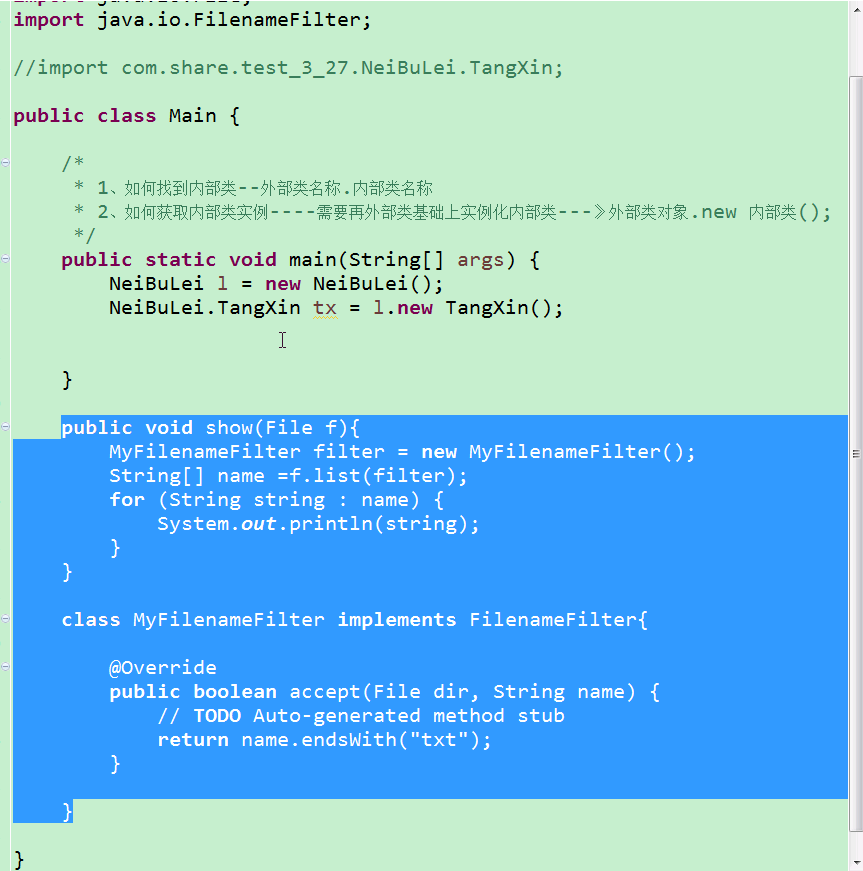
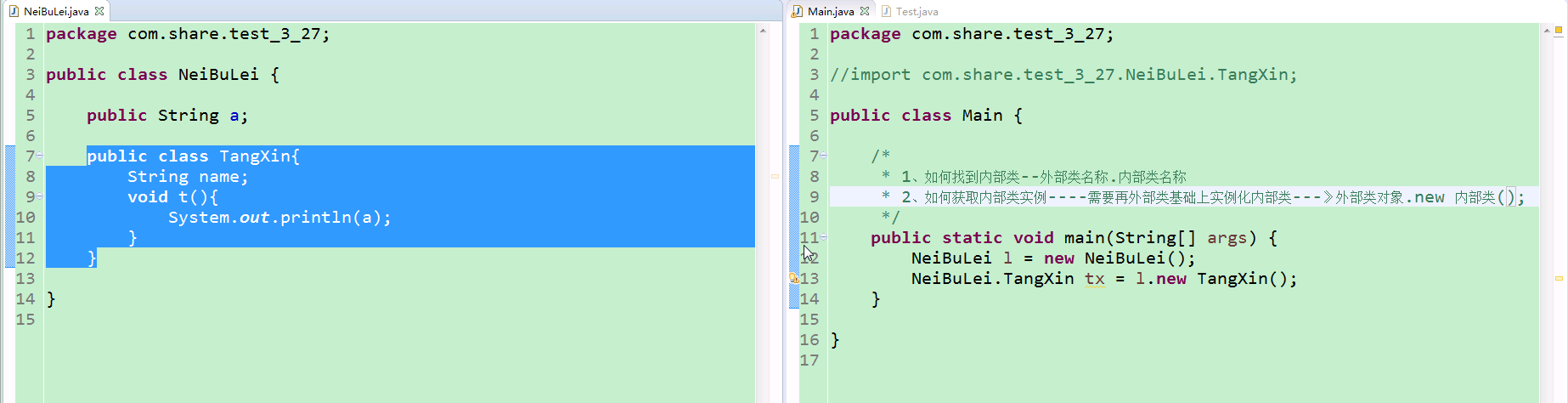
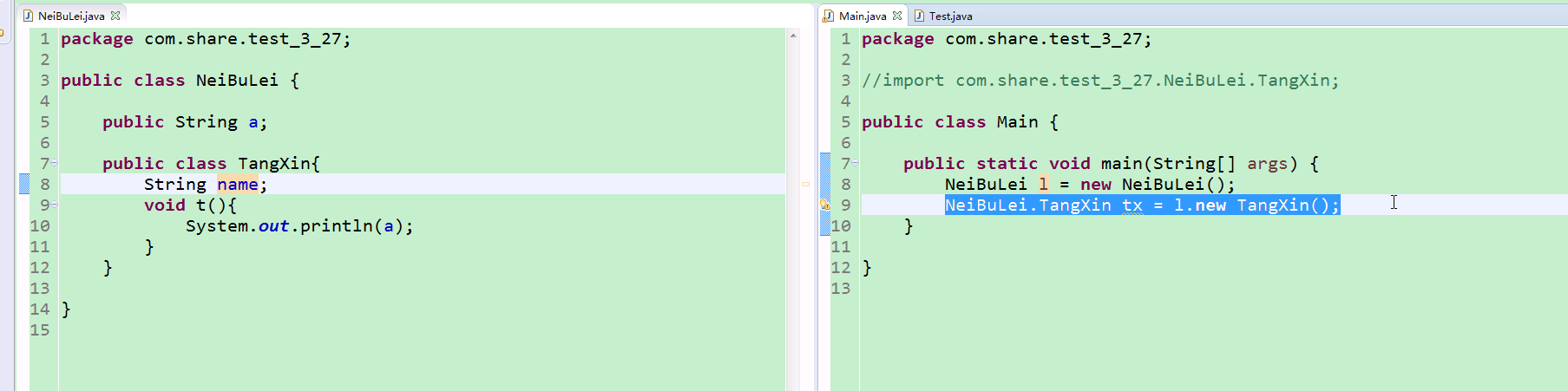
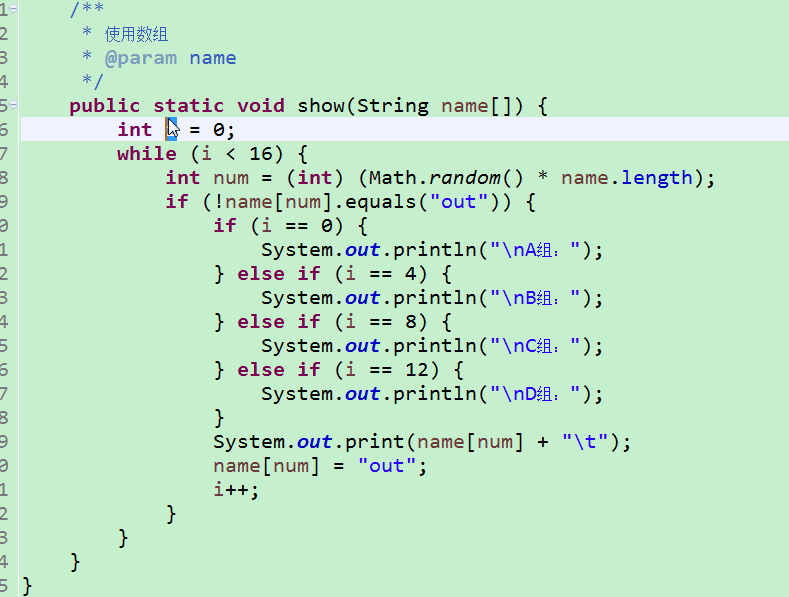
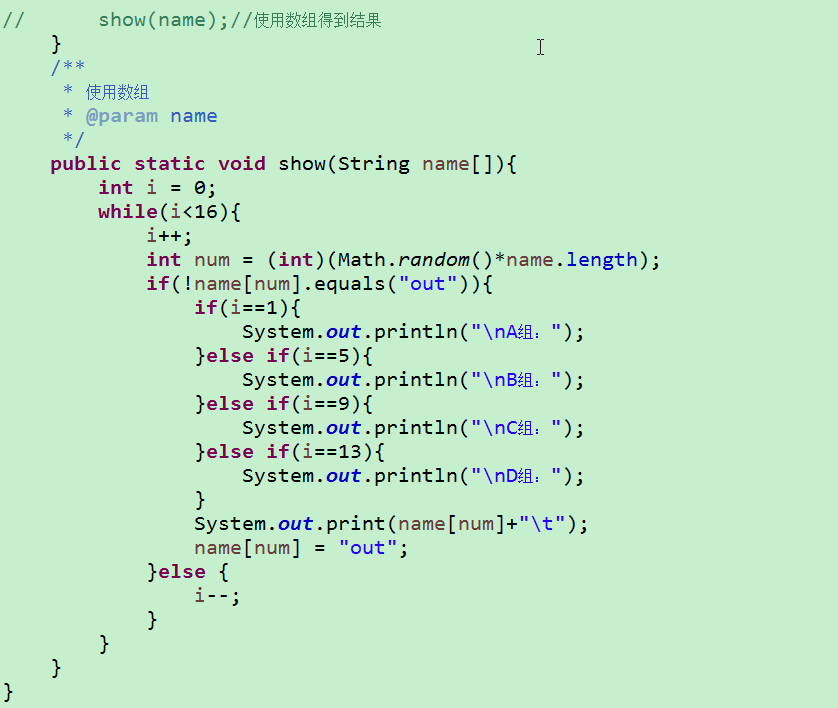
实例1:
写一个方法,显示文件夹下所有的.apk文件
* 要求在查找方法内部使用局部内部类
思路:
局部内部类就是创建过滤器类实现文件名过滤器重写过滤方法
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.share.demo03_28;
import java.io.File; import java.io.FilenameFilter;
public class FindFile { public static void main(String[] args) { find(); }
public static void find() { class FileApkFilter implements FilenameFilter {
@Override public boolean accept(File dir, String name) {
return name.endsWith(".apk"); }
} File file = new File("E:\\"); if (file.isDirectory()) { File[] fa = file.listFiles(new FileApkFilter()); for (File f1 : fa) { System.out.println(f1.getName()); } } System.out.println(file.getName()); } }
|
实例2:
分别使用局部内部类和匿名内部类来实现list集合中自定义类的排序
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84
| package com.share.demo03_28;
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List;
public class SortFile { public static void main(String[] args) { sort(); sort1(); }
public static void sort() { List<Person> list = new ArrayList<Person>();
Person p1 = new Person("p1", 11); Person p2 = new Person("p2", 12); Person p3 = new Person("p3", 13); Person p4 = new Person("p4", 14);
list.add(p4); list.add(p2); list.add(p1); list.add(p3);
Collections.sort(list, new Comparator<Person>() {
@Override public int compare(Person o1, Person o2) { if (!o1.getName().equals(o2.getName())) { return o1.getName().compareTo(o2.getName()); } else if (o1.getAge() != o2.getAge()) { return o1.getAge() - o2.getAge(); } return 0; }
}); System.out.println(list); }
public static void sort1() { class MyComparator implements Comparator<Person> {
@Override public int compare(Person o1, Person o2) { if (!o1.getName().equals(o2.getName())) { return o1.getName().compareTo(o2.getName()); } else if (o1.getAge() != o2.getAge()) { return o1.getAge() - o2.getAge(); } return 0; }
}
List<Person> list = new ArrayList<Person>();
Person p1 = new Person("p1", 11); Person p2 = new Person("p2", 12); Person p3 = new Person("p3", 13); Person p4 = new Person("p4", 14);
list.add(p4); list.add(p2); list.add(p1); list.add(p3); Collections.sort(list, new MyComparator()); System.out.println(list); }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| package com.share.demo03_28;
public class Person { private String name; private int age;
public Person() {
}
public Person(String name, int age) { this.name = name; this.age = age; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
@Override public String toString() { return "name: "+name+" age: "+age; } }
|
知识点2:
IO流
输入流,输出流
字节流,字符流
节点流,处理流
实例2:
将文件读取到程序内,并在控制台上现实打印
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74
| package com.share.demo03_28;
import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.util.Arrays;
public class InputStreamTest { public static void main(String[] args) { test1(); } public static void test(){ File file=new File("D:\\1myDocFile\\20170328\\Test.java"); InputStream is=null; try { is=new FileInputStream(file); int len=0; try { while((len=is.read())!=-1){ System.out.print((char)len); } } catch (IOException e) { e.printStackTrace(); } } catch (FileNotFoundException e) { e.printStackTrace(); }finally{ if(is!=null){ try { is.close(); } catch (IOException e) { e.printStackTrace(); } } } } public static void test1(){ File file=new File("D:\\1myDocFile\\20170328\\Test.java"); InputStream is=null; try { is=new FileInputStream(file); byte[] b=new byte[1024*3]; try { int len=is.read(b); System.out.println(len); System.out.println(Arrays.toString(b)); for(byte b1:b){ System.out.print((char)b1); } } catch (IOException e) { e.printStackTrace(); } } catch (FileNotFoundException e) { e.printStackTrace(); }finally{ if(is!=null){ try { is.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
|
实例3:
将一个文件夹下的一文件复制到另一个文件夹下面
思路:
IO流的组合使用,将读进内存的内容写入到另一个文件中。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
| package com.share.demo03_28;
import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream;
public class OutputStreamTest { public static void main(String[] args) { write(); }
public static void write() { File srcFile = new File("D:\\1myDocFile\\20170328\\Test.java"); File objFile = new File("C:\\Users\\Administrator\\Desktop\\T.java"); copy(srcFile, objFile); }
public static void copy(File srcFile, File objFile) { InputStream is = null; OutputStream os = null; try { is = new FileInputStream(srcFile); os = new FileOutputStream(objFile); int i = 0; while ((i = is.read()) != -1) { os.write(i); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally {
try { if (is != null) is.close(); if(os!=null){ os.flush(); os.close(); } } catch (IOException e) { e.printStackTrace(); }
}
}
}
|
实例4:
从键盘输入一行字符串,然后将这个字符串存进一个文件中。
思路:
将字符串转换成字符数组或者将字符串直接转换成byte数组
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public static void test() { Scanner sc = new Scanner(System.in); System.out.println("请输入一行数据:"); String s = sc.nextLine(); char[] c = s.toCharArray();
OutputStream os = null; try { os = new FileOutputStream(new File("C:\\Users\\Administrator\\Desktop\\T.txt")); os.write(s.getBytes());
} catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }
}
|
实例5:
遍历map
思路:
1.通过key的set找出value的set
2.调用map的entrySet方法
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| package com.share.demo03_28;
import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Map.Entry;
public class MapTraverTest { public static void main(String[] args) { test(); }
public static void test() { Map<String, String> map = new HashMap<String, String>(); map.put("one", "1"); map.put("two", "2"); map.put("three", "3"); map.put("four", "4");
Iterator<Entry<String, String>> it = map.entrySet().iterator(); Iterator<String> it1 = map.keySet().iterator(); Iterator<String> it2 = map.values().iterator();
while (it.hasNext()) { System.out.println(it.next()); } System.out.println("---------------------");
while (it1.hasNext()) { String s = it1.next(); System.out.println(s + "=" + map.get(s)); } while (it2.hasNext()) { System.out.println(it2.next()); } System.out.println(map); System.out.println(map.entrySet()); } }
|
实例6:
从键盘输入一行字符串,直到输入quit则退出输入,
将输入的所有字符串写入到文件中。
思路:
死循环输出直到输入为quit就退出
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| public static void test() { Scanner sc = new Scanner(System.in); StringBuffer sb =null; String s=""; OutputStream os = null; try { os = new FileOutputStream(new File("C:\\Users\\Administrator\\Desktop\\T.txt"),true); System.out.println("请输入一行字符串:(输入quit则退出)"); sb=new StringBuffer();
String str=""; do{ sb.append(str); str=sc.nextLine(); }while(!str.equals("quit"));
s=sb.toString(); os.write(s.getBytes());
} catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }
}
|
实例7:
将一个文件夹下的所有文件复制到另一个文件夹下面,如果源文件夹的源文件不为空,那么复制后的文件也不为空
思路:
通过递归将所有的文件夹创建出来,再从最底层开始创建文件,再将源文件的文件通过输入流读到内存中,再将文件内容输出到目标文件中。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| public static void copyDirctory(){ File srcFile=new File("D:\\1myDocFile"); File objFile=new File("C:\\Users\\Administrator\\Desktop"); runCopy(srcFile,objFile); } public static void runCopy(File srcFile,File objFile){ if(srcFile.isDirectory()){ objFile=new File(objFile,srcFile.getName()); objFile.mkdirs(); File[] childFile=srcFile.listFiles(); for(File f:childFile){ runCopy(f, objFile); } }else{ InputStream is=null; OutputStream os=null; try { objFile=new File(objFile,srcFile.getName()); is=new FileInputStream(srcFile); os=new FileOutputStream(objFile); int len=0; byte[] b=new byte[1024]; while((len=is.read(b))!=-1){ os.write(b, 0, len); } } catch (IOException e) { e.printStackTrace(); }finally{ try { if(is!=null) is.close(); if(os!=null){ os.flush(); os.close(); } } catch (IOException e) { e.printStackTrace(); } } } }
|