2017-03-29-IO流
知识点1:
reader
writer
FileReader
FileWriter
知识点2:
获取当前时间:System.currentTimeMillis();
获取运行时间,两次获取时间的时间差
格式化时间:
1 2 3 4
| Date date=new Date(System.currentTimeMillis()); SimpleDateFormat sdf=new SimpleDateFormat("YYYY-MM-dd HH:mm:ss"); String str=sdf.format(date); System.out.println("当前日期:"+str);
|
知识点3:
获取字符编码:
System.getProperty(“file.encoding”);
字节字符转换流
知识点4:
流的概念的产生
程序和其他数据存储介质进行数据交换的目的产生了流,
流是一种数据通道,有三个基本维度(出入,数据传输单位,功能)
抽象的流只有两个维度(出入,数据传输单位),有第三个维度才能成为一个具体实际的流
除了功能维度分为节点和处理,实际的维度还可以细分特殊专业化(文件,缓冲,转换,数据,打印,对象)
其实功能维度可以理解为连接的对象的不同的维度,直接连接数据源的流是节点流,通过对节点流进行封装和处理的流是处理流
具体的维度则可以看成是真正的功能维度(文件,缓冲,转换,数据,打印,对象)
输入流的方法是读,读一个字节和读一个字符的区别
输出流的方法是写,写一个字节和写一个字符的区别
图文详解:
数据流:
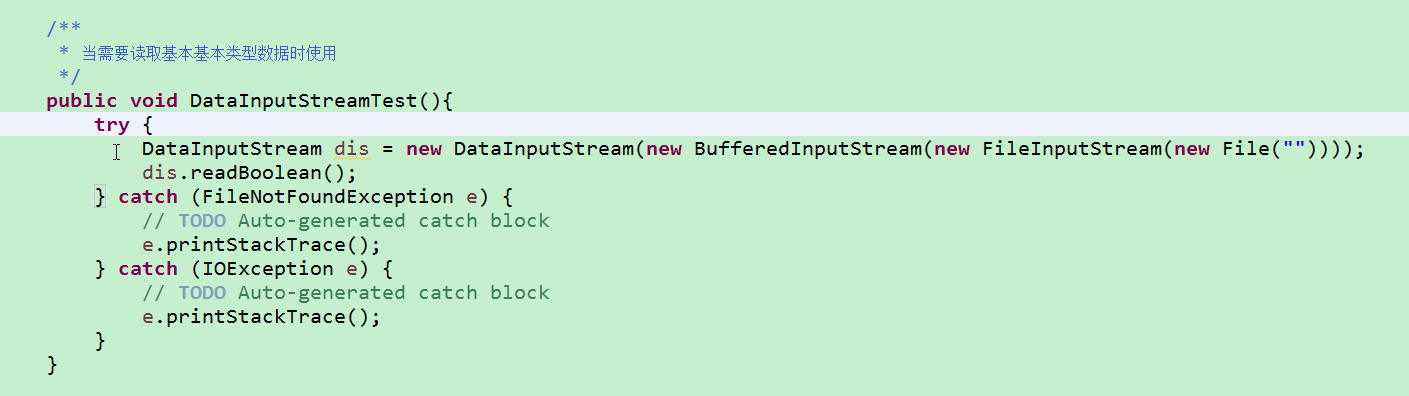
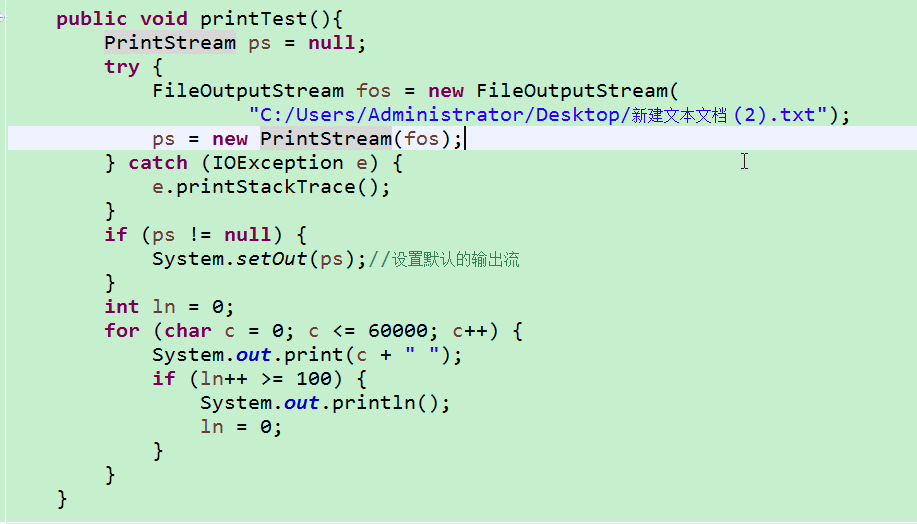
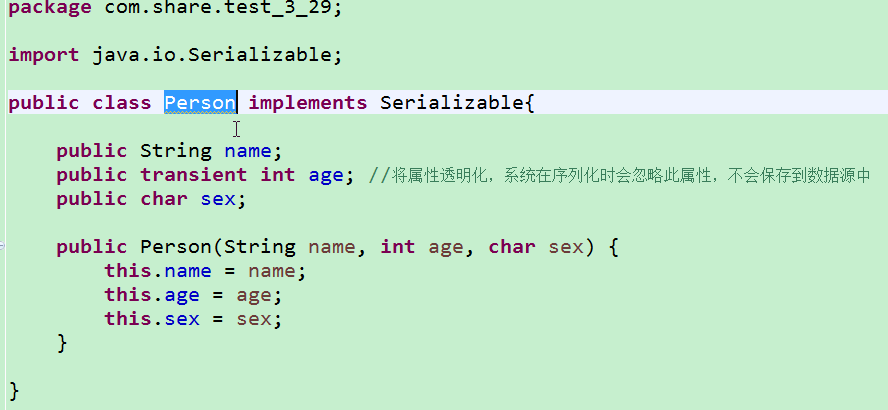
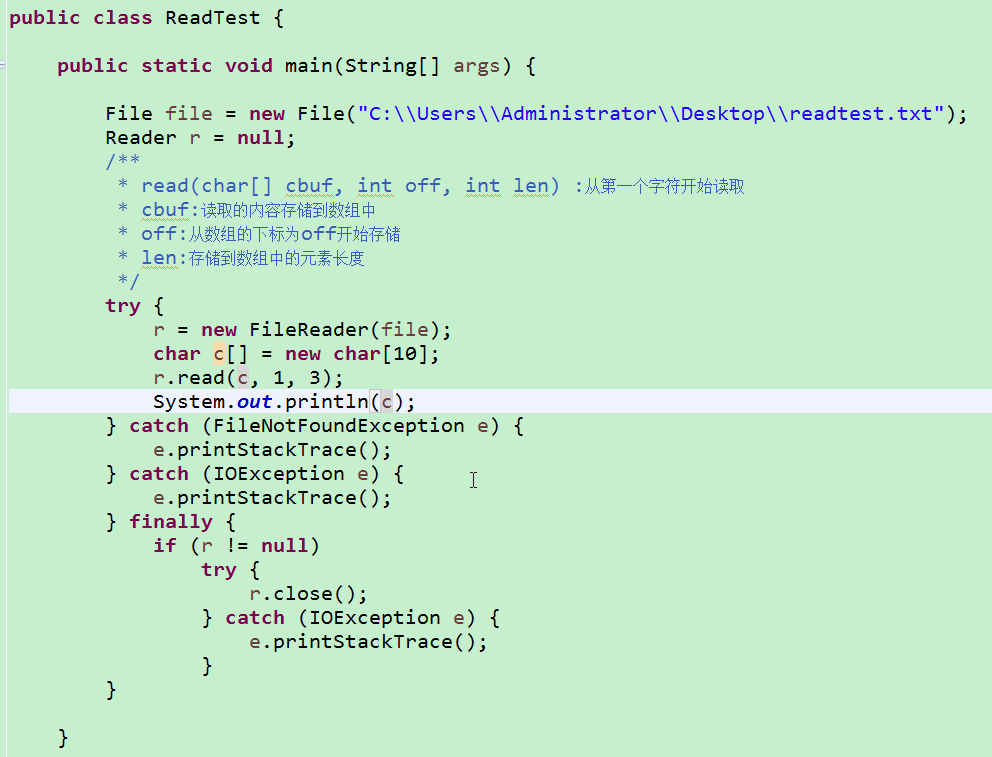
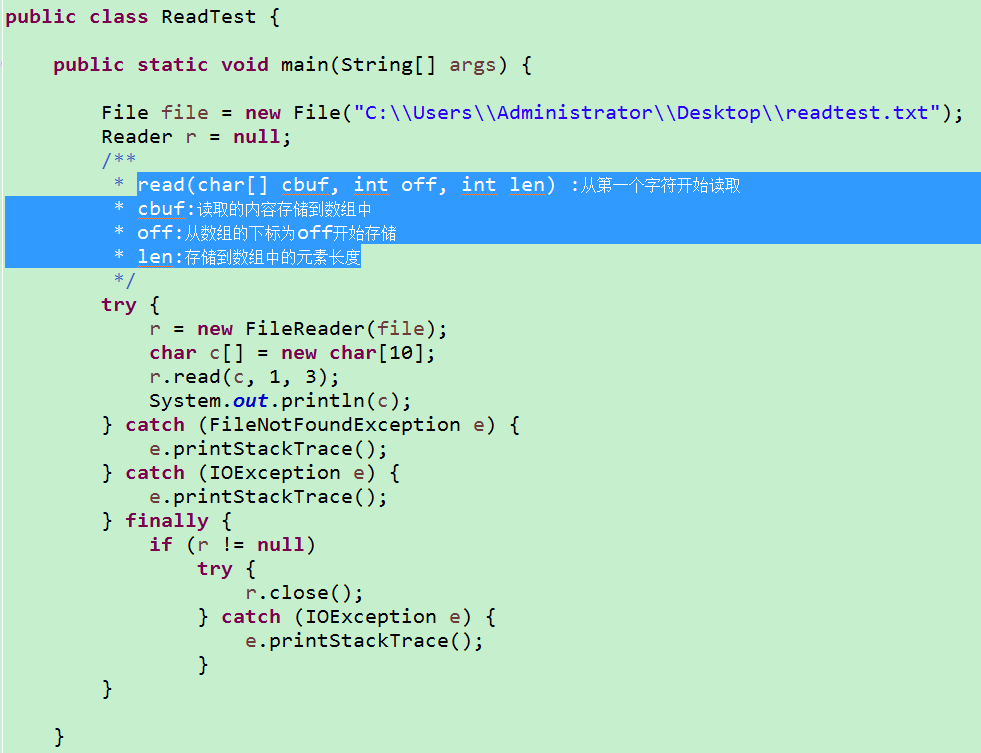

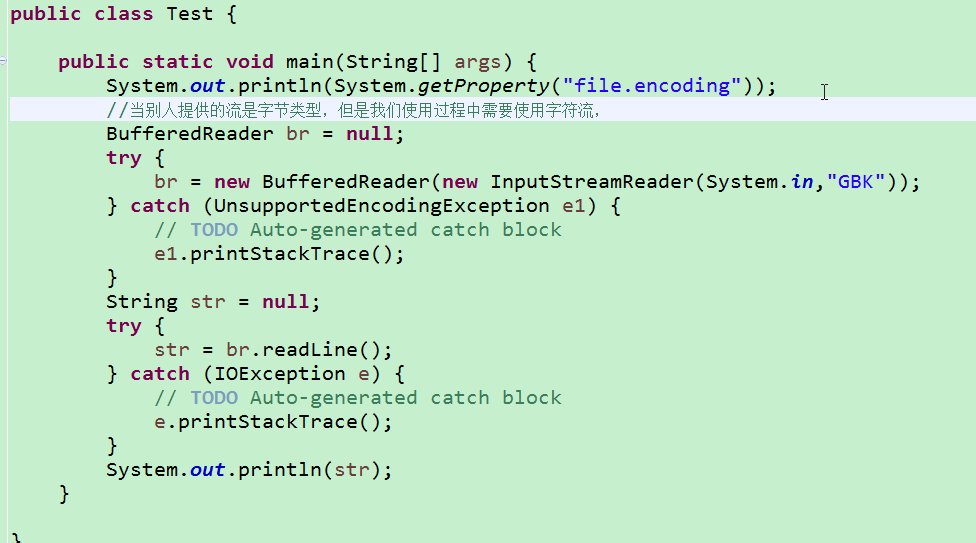

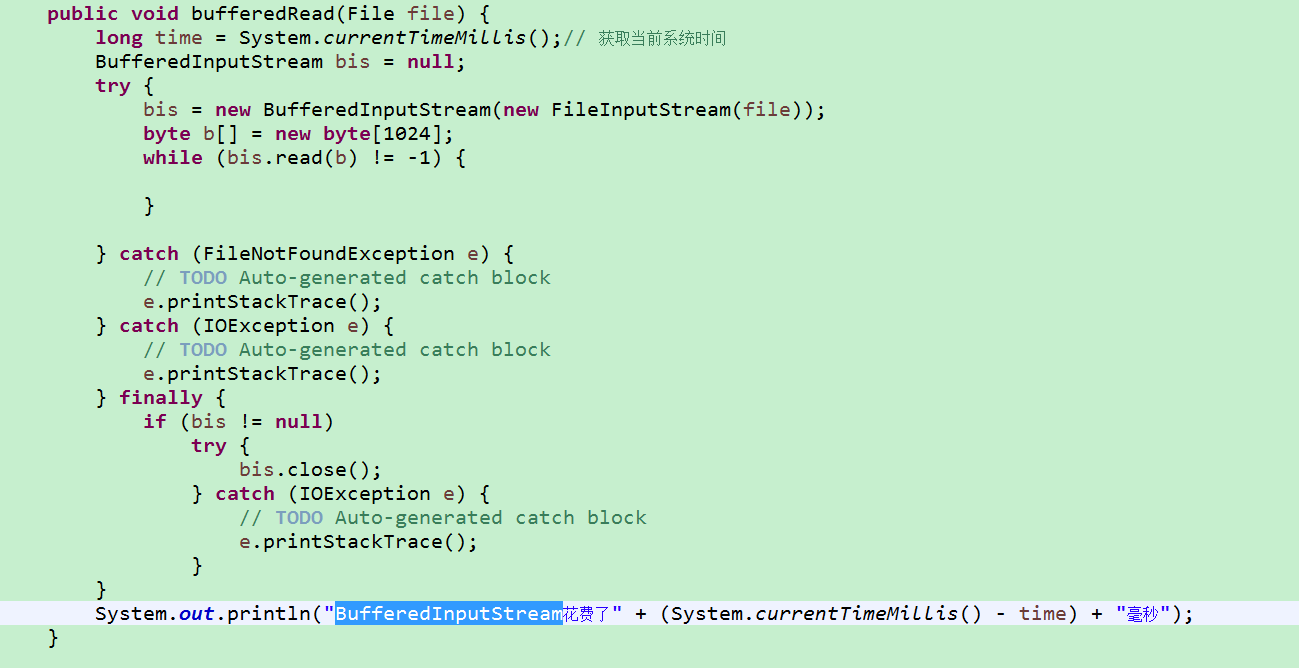
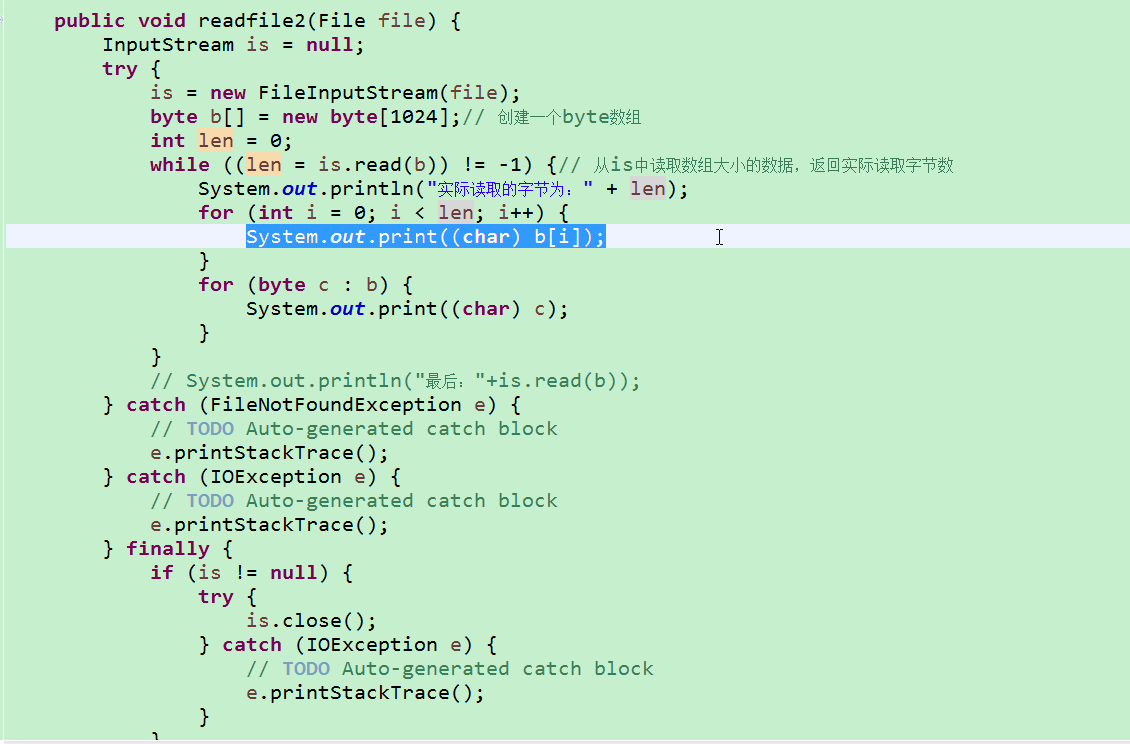
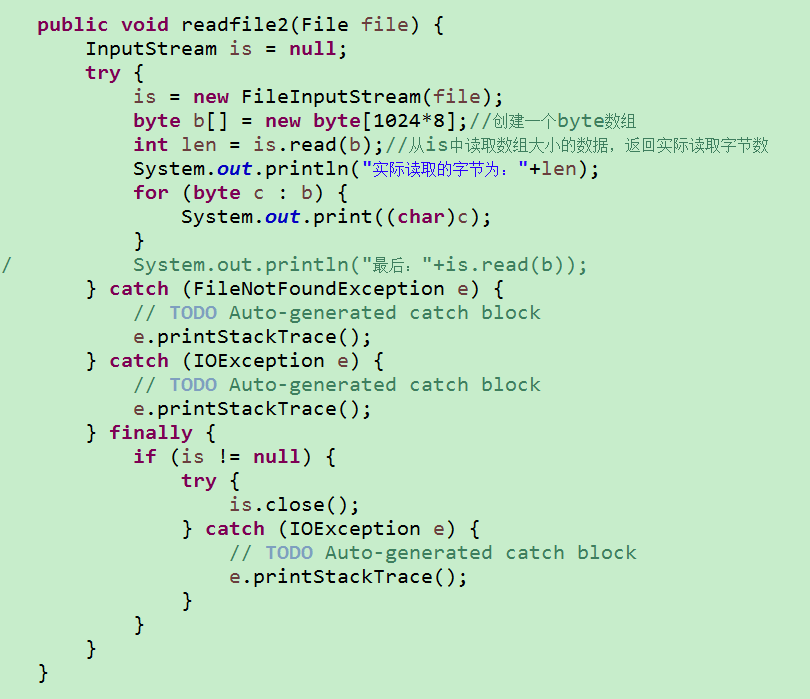
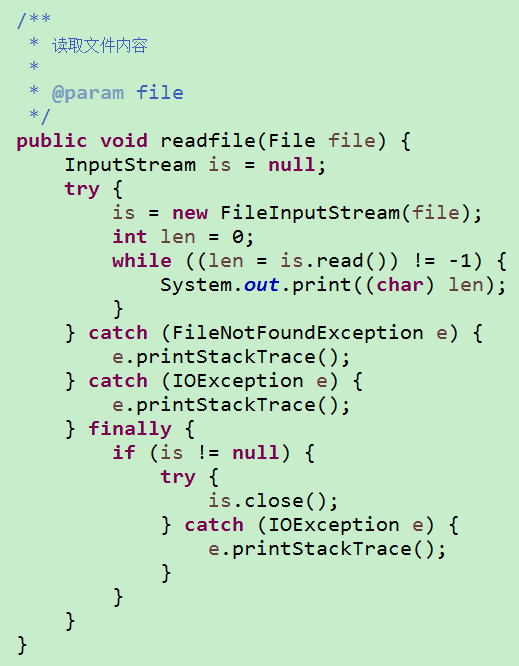
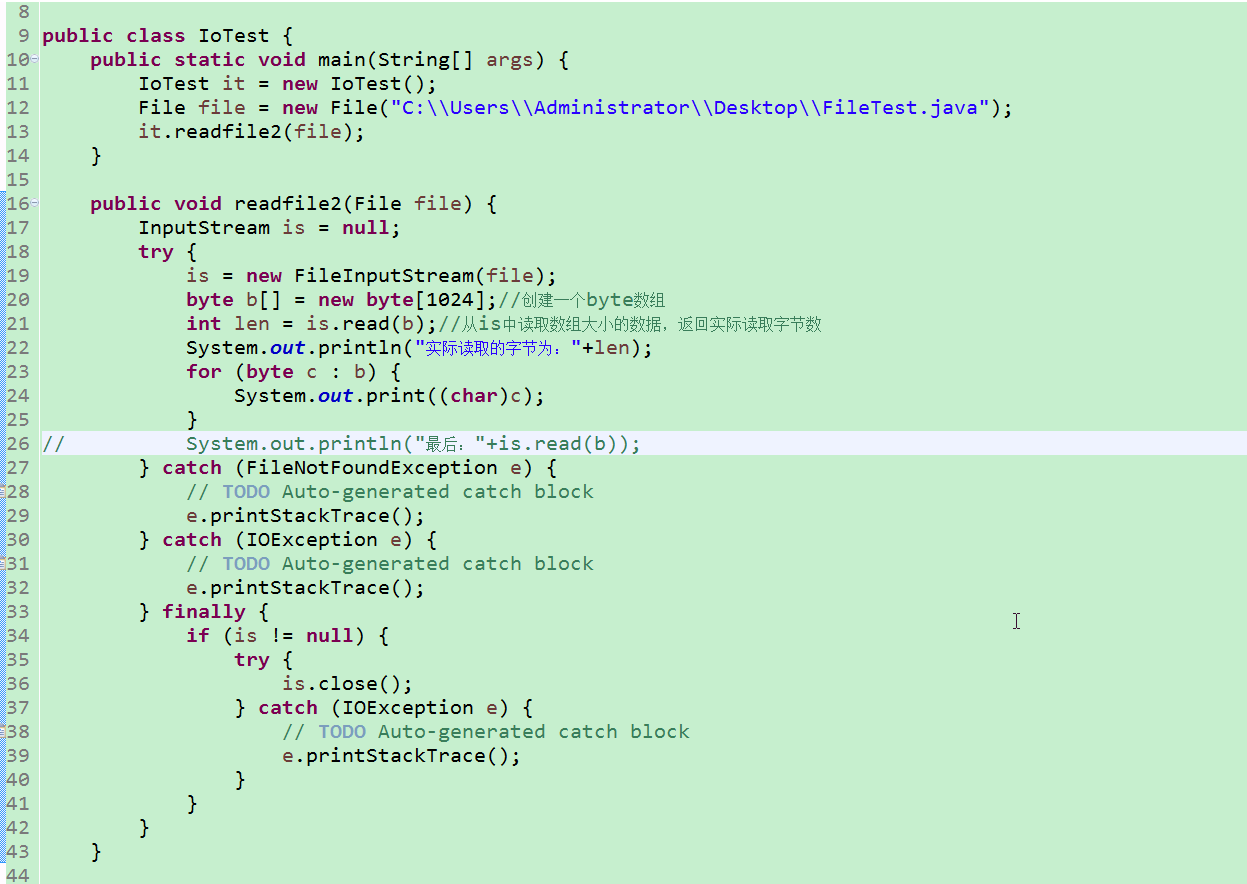
其他1:
其他2:
其他3:
其他4:
其他5:
其他6:
其他7:
其他8:
其他9:
其他10:
其他11:
其他:
其他:
实例1:
将一个文件中的内容复制到另一个文件中(使用字符流)
思路:
FileReader
FileWriter
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
| package com.share.demo03_29_IO流;
import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.Reader; import java.io.Writer;
public class Test { public static void main(String[] args) { test(); } public static void test(){ File src=new File("C:\\Users\\Administrator\\Desktop\\惩罚游戏.txt"); File obj=new File("D:\\惩罚游戏.txt"); Reader reader=null; Writer writer=null; try { File f=new File(obj.getParent()); f.mkdirs(); obj.createNewFile(); reader=new FileReader(src); writer=new FileWriter(obj,true); char[] cbuf=new char[1024]; int len=0; while((len=reader.read(cbuf))!=-1){ writer.write(cbuf, 0, len); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ try { if(reader!=null) reader.close(); if(writer!=null){ writer.flush(); writer.close(); } } catch (IOException e) { e.printStackTrace(); } } } }
|
实例2:
使用BufferedInputStream,BufferedOutputStream来实现文件复制
思路:
使用即可
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| public static void test2() { File src = new File("C:\\Users\\Administrator\\Desktop\\惩罚游戏.txt"); File obj = new File("D:\\惩罚游戏.txt");
InputStream is = null; OutputStream os = null;
BufferedInputStream bis = null; BufferedOutputStream bos = null;
try { is = new FileInputStream(src); os = new FileOutputStream(obj,true);
bis = new BufferedInputStream(is); bos = new BufferedOutputStream(os);
byte[] b = new byte[1024]; int len = 0;
while ((len = bis.read(b)) != -1) { bos.write(b, 0, len); }
} catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { try { if (bis != null) { bis.close(); } if (bos != null) { bos.flush(); bos.close(); } } catch (IOException e) { e.printStackTrace(); } }
}
|
实例3:
实现文件剪切
思路:
先复制后删除
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| public static void test4(){ File src=new File("C:\\Users\\Administrator\\Desktop\\jdk-8u121-docs-all"); File obj=new File("F:\\"); cutFile(src,obj); } public static void cutFile(File src,File obj){ obj=new File(obj,src.getName()); if(src.isDirectory()){ obj.mkdirs(); File[] fl=src.listFiles(); for(File f:fl){ cutFile(f,obj); } }else{ BufferedInputStream bis=null; BufferedOutputStream bos=null; try { obj.createNewFile(); bis=new BufferedInputStream(new FileInputStream(src)); bos=new BufferedOutputStream(new FileOutputStream(obj)); byte[] b=new byte[1024]; int len=0; while((len=bis.read(b))!=-1){ bos.write(b, 0, len); } } catch (IOException e) { e.printStackTrace(); }finally{ try { if(bis!=null) bis.close(); if(bos!=null){ bos.flush(); bos.close(); } } catch (IOException e) { e.printStackTrace(); } } } src.delete(); }
|
实例4:
将对象通过对象流存储进入文件中,再通过对象流将文件中存储的对象取出来
思路:
重点是将对象序列化,对象的类实现序列化接口Serializabl
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.share.demo03_29_IO流;
import java.io.Serializable;
public class Person implements Serializable{
private static final long serialVersionUID = -1032882906825208167L; public String name; public int age; public char sex; public Person(String name, int age, char sex) { super(); this.name = name; this.age = age; this.sex = sex; } @Override public String toString() { return "姓名:"+name+" 年龄:"+age+" 性别:"+sex; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109
| package com.share.demo03_29_IO流;
import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.util.Scanner;
public class ObjectStreamTest { public static void main(String[] args) { start(); }
public static void start() { Scanner sc = new Scanner(System.in); System.out.println("选择:1.读档,2.新游戏"); String str = sc.next(); Person p=null; switch (str) { case "1": p=load(); System.out.println(p); break;
default: p = creatRole(); save(p); System.out.println("存储成功"); break; } }
public static Person creatRole() { Scanner sc = new Scanner(System.in); System.out.println("请输入名字:"); String name = sc.next(); System.out.println("请输入年龄:"); int age = sc.nextInt(); System.out.println("请输入性别:"); char sex = sc.next().charAt(0);
return new Person(name, age, sex); }
public static void save(Person p) { File file = new File("C:\\Users\\Administrator\\Desktop\\T.txt"); ObjectOutputStream oos = null;
try { oos = new ObjectOutputStream(new FileOutputStream(file)); oos.writeObject(p); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { try { if (oos != null) { oos.flush(); oos.close(); } } catch (IOException e) { e.printStackTrace(); } } }
public static Person load() { Person p = null; File file = new File("C:\\Users\\Administrator\\Desktop\\T.txt"); ObjectInputStream ois = null;
try { ois = new ObjectInputStream(new FileInputStream(file)); p = (Person) ois.readObject(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } finally { try { if (ois != null) ois.close(); } catch (IOException e) { e.printStackTrace(); } } return p; }
}
|
实例5:
使用打印输出字符流和打印输出字节流,实现输出信息保存到文件中。
思路:
使用PrintStream和PrintWriter来实现。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| package com.share.demo03_29_IO流;
import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.PrintStream;
public class PrintStreamTest { public static void main(String[] args) { test(); }
public static void test() { File file = new File("C:\\Users\\Administrator\\Desktop\\T.txt");
PrintStream ps = null;
try { ps = new PrintStream(new FileOutputStream(file)); } catch (FileNotFoundException e) { e.printStackTrace(); } if(ps!=null){ System.setOut(ps); } int count=0; for(int i=-1;i<123;i++){ System.out.print((char)i+"\t"); if(count==10){ count=0; System.out.println(); } count++; } }
}
|