2017-04-10-设计模式
知识点1:
单例模式
单个实例
单线程中只有一个实例;
多线程中需要加锁确定只有一个实例,但是加锁又要区分很多情况。
单例模式:一个类的对象不能直接通过构造方法创建,因为构造方法是私有的,通过另外的方法来创建实例,同时在这个方法中控制这个只调用了一次构造方法来创建实例,如果对象已经创建就直接返回已经创建的对象,如果还没有创建这个对象那就创建这个对象。
一个类A,构造方法的属性私有,在类中有一个类A的对象作为成员属性,如果类A的对象为空,在另一个方法中得到调用构造方法创建对象并把对象赋值给类A的成员属性。,
最简单的单例模式:
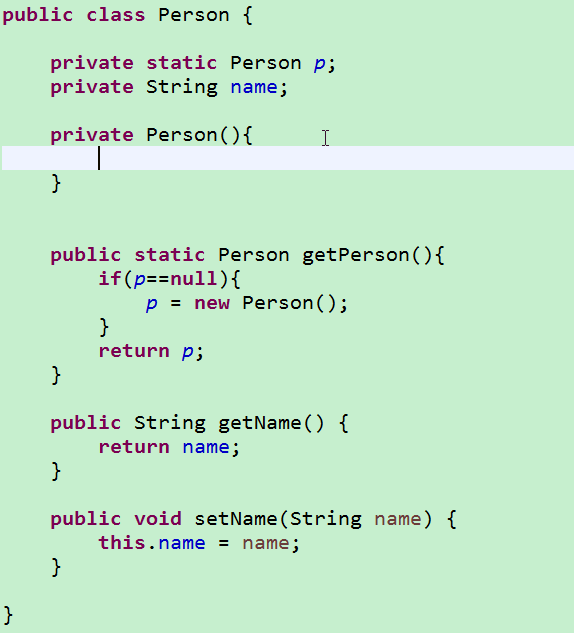
知识点2:
代理模式
两个有相同方法的类A、B(可以是一个类对另一个类的继承,或者是继承同一父类,或者是实现相同的接口),B类中有A类的成员,B类的方法实际是用A类的对象调用A类的相同方法。
知识点3:
工厂模式
一个抽象类A有一种抽象的方法method(),抽象类的子类a、b、c、d是一系列的具体的方法method()的类,每个子类中的方法的具体实现不同,再创建一个工厂类,根据不同的条件创建不同的子类,声明的引用是父类的引用,实际的对象是子类的。
通过工厂类的创造方法来创建某个类的具体的子类的对象,具体是根据传入的参数来判断。
知识点4:
观察者模式(这种观察者模式理解有问题,具体的正确的模式参考百度结果)
观察者类中有被观察的对象和需要通知的类作为成员,需要通知的类的对象可以是多个所以一般存储进入集合中,添加通知的成员和移除通知的成员的方法,事件发生后调用通知方法。
实例1:
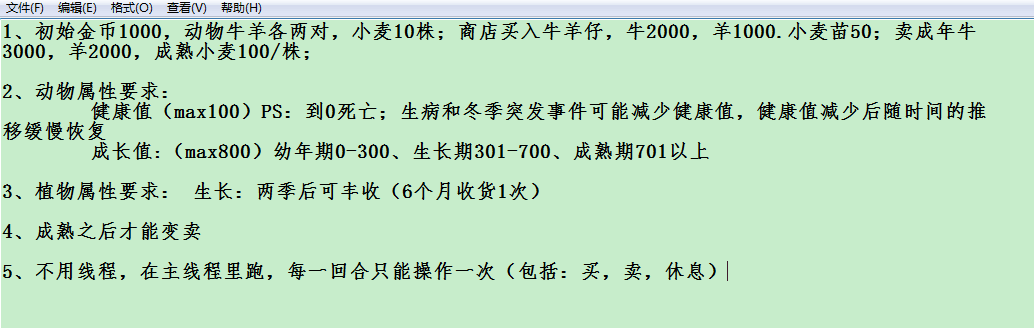
知识点1实例:
写一个简单的单例模式的类,并得到对象然后检测创建的多个类的对象是否是同一对象
思路:
直接写出单例模式即可
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class SimpleSingle { private static SimpleSingle single=null; private SimpleSingle(){ } public static SimpleSingle getInstance(){ if(single==null){ single=new SimpleSingle(); } return single; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class SingletonTest { public static void main(String[] args) { SimpleSingle single1=SimpleSingle.getInstance(); SimpleSingle single2=SimpleSingle.getInstance(); SimpleSingle single3=SimpleSingle.getInstance(); System.out.println(single1); System.out.println(single2); System.out.println(single3); System.out.println(single1==single2); System.out.println(single1==single3); System.out.println(single2==single3); } }
|
知识点2实例:
写一个代理模式的例子
思路:
A类和B类(是代理类)具有相同的方法(B继承A,或者A和B继承同一个类,或者A和B实现同一接口),B类中有A类的实例,B类中的方法实现,都是通过A类对象的方法实现。
具体例子:男生给女生送礼物,不直接送,通过小孩来帮忙送礼物,女生从小孩那里得到男生的礼物。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| package com.share.eg02代理模式;
public class ProxyTest { public static void main(String[] args) { Kid kid=new Kid("kid"); Girl girl=new Girl("girl"); girl.receiveGift(kid.sendGift()); } }
|
1 2 3 4 5 6 7 8 9 10
| package com.share.eg02代理模式;
public interface SendGift { public Gift sendGift(); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| package com.share.eg02代理模式;
public class Boy implements SendGift{ private String name; public Boy() { super(); } public Boy(String name) { super(); this.name=name; } public String getName() { return name; }
public void setName(String name) { this.name = name; }
@Override public Gift sendGift() { Gift gift=new Gift(name+"送出的礼物"); System.out.println(name+"送出了礼物"); return gift; }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| package com.share.eg02代理模式;
public class Kid implements SendGift { private Boy boy; private String name; public Kid() { super(); boy=new Boy(); }
public Kid(String name) { super(); this.name = name; boy=new Boy("boy"); }
public Kid(Boy boy, String name) { super(); this.boy = boy; this.name = name; }
public Boy getBoy() { return boy; }
public void setBoy(Boy boy) { this.boy = boy; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
@Override public Gift sendGift() { System.out.println(name+"转交了"+boy.getName()+"的礼物"); return boy.sendGift(); }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package com.share.eg02代理模式;
public class Girl { private String name;
public Girl() { super(); }
public Girl(String name) { super(); this.name = name; }
public String getName() { return name; }
public void setName(String name) { this.name = name; } public void receiveGift(Gift gift){ System.out.println(name+"收到了"+gift.getName()); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.share.eg02代理模式;
public class Gift { private String name;
public Gift() { super(); }
public Gift(String name) { super(); this.name = name; }
public String getName() { return name; }
public void setName(String name) { this.name = name; } }
|
知识点3实例:
写一个工厂模式的例子
思路:
一个抽象的类A,一些继承自A的子类a、b、c、d对A中的一个抽象方法进行了不同的重写和实现,一个A的对象的工厂类,根据不同的条件创建A的子类的对象并返回。
实际例子
运算抽象类Operation,其子类分别为加减乘除的实现类,运算工厂类的方法根据参数判断返回相应的子类。
核心代码:
package com.share.eg03工厂模式;
1 2 3 4 5 6 7 8 9 10 11 12 13 14
|
public class FactoryTest { public static void main(String[] args) { OperationFactory factory=new OperationFactory(); Operation operation=factory.creatOperation("+"); operation.setOne(10); operation.setTwo(2); System.out.println(operation.getResult()); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| package com.share.eg03工厂模式;
public abstract class Operation { private double one; private double two; public Operation() { super(); } public Operation(double one, double two) { super(); this.one = one; this.two = two; } public double getOne() { return one; } public void setOne(double one) { this.one = one; } public double getTwo() { return two; } public void setTwo(double two) { this.two = two; } public abstract double getResult(); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package com.share.eg03工厂模式;
public class OperationFactory { public Operation creatOperation(String str){ Operation operation=null; switch (str) { case "+": operation=new AddOperation(); break; case "-": operation=new SubOperation(); break; case "*": operation=new MulitOperation(); break; case "/": operation=new DiviOperation(); break;
default: operation=new AddOperation(); break; } return operation; } }
|
1 2 3 4 5 6 7 8 9 10 11
| package com.share.eg03工厂模式;
public class AddOperation extends Operation {
@Override public double getResult() { return getOne()+getTwo(); }
}
|
1 2 3 4 5 6 7 8 9 10 11
| package com.share.eg03工厂模式;
public class SubOperation extends Operation{
@Override public double getResult() { return getOne()-getTwo(); }
}
|
1 2 3 4 5 6 7 8 9 10 11
| package com.share.eg03工厂模式;
public class MulitOperation extends Operation{
@Override public double getResult() { return getOne()*getTwo(); }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package com.share.eg03工厂模式;
public class DiviOperation extends Operation{
@Override public double getResult() { double result=0; try { result=getOne()/getTwo(); } catch (Exception e) { System.out.println("除数不能为0"); } return result; }
}
|
知识点4实例:
写一个观察者模式的例子
观察者功能:通知和观察,观察者类中有要通知的对象和被观察的对象,当被观察的对象的某些事件发生时,观察者马上通知要通知的对象。
实际例子:
员工上班玩耍,老板回来了,前台妹子提醒正在玩游戏的员工
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13
| package com.share.eg04观察者模式;
public class ObserverTest { public static void main(String[] args) { Observer ob=new Observer(); Employee e=new Employee(); e.play(); ob.addEmployee(e); ob.somethingHappened(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| package com.share.eg04观察者模式;
import java.util.ArrayList; import java.util.List;
public class Observer { private List<Employee> employees; private Boss boss; public Observer() { super(); employees=new ArrayList<Employee>(); boss=new Boss(); } public Observer(List<Employee> employee, Boss boss) { super(); this.employees = employee; this.boss = boss; } public List<Employee> getEmployee() { return employees; } public void setEmployee(List<Employee> employee) { this.employees = employee; } public Boss getBoss() { return boss; } public void setBoss(Boss boss) { this.boss = boss; } public void inform(){ System.out.println("快回去工作"); for(Employee e:employees){ e.work(); } } public void somethingHappened(){ boss.comeBack(); inform(); } public void addEmployee(Employee e){ employees.add(e); } }
|
1 2 3 4 5 6 7 8 9 10
| package com.share.eg04观察者模式;
public class Employee { public void play(){ System.out.println("employee 在玩"); } public void work(){ System.out.println("employee 在工作"); } }
|
1 2 3 4 5 6 7
| package com.share.eg04观察者模式;
public class Boss { public void comeBack(){ System.out.println("boss 回来了"); } }
|