2017-04-11-反射-JSON解析
知识点1:
反射的概念
反射中的类
Class 是类的抽象
类是对象的抽象
Class类的对象就是一个类(模板,设计图)
反射的作用:
将类中所有属性和方法(包括构造方法)都提取出来。
获取Class对象来存储类(Class类很特殊,没有构造方法,不能创建它依附于其他类,是一个类的抽象,类的类)
获取Class对象的三种方法:
1、类名.class
2、对象.getClass();获取的是对象的实际类型,多态中运行时动态获取对象类型
3、Class.forName(类的路径及名称);
Class 类对象主要的方法有5个方面:
1、提取构造方法 getConstructor()
2、提取方法getMethod()
3、提取属性getField()
4、提取父类getSuperclass()
5、创建类的对象(实际是调用类的无参公有构造方法,可以将这个功能划分到提取构造方法里面去)
具体的使用参见例子
基本数据类型也可以使用类似的类名.class
例如:int.class
知识点2:
JSON解析
json:JavaScript Object Notation JavaScript 对象标记
json是一种数据存储格式,存储形式如Java中的Map一样通过key-value这样的键值对来存储
由于Map的这种key-value映射和类的属性名和属性值的功能相似,于是就可以将这种键值对的映射转换为对象进行存储。
存储数据的格式
map
json
xml
json和xml都要解析才能够使用
json解析就是将json形式的数据通过类的对象的方式存储起来,再利用类的对象的属性得到相应的值。
json解析要使用到Gson的jar包
具体解析参考示例
实例1:
反射的相关操作
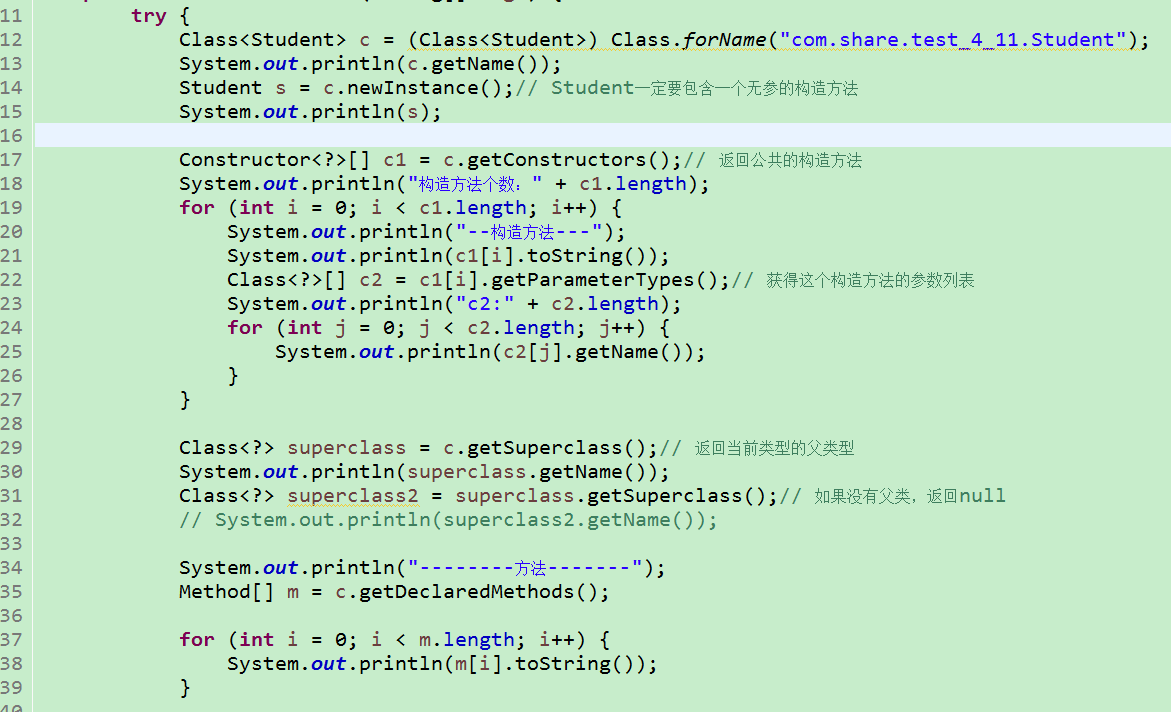
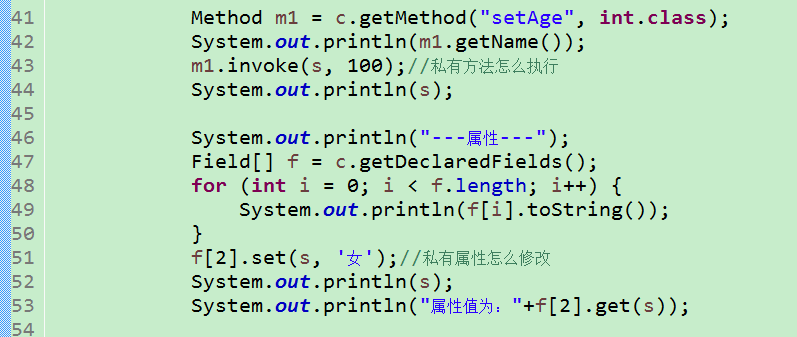
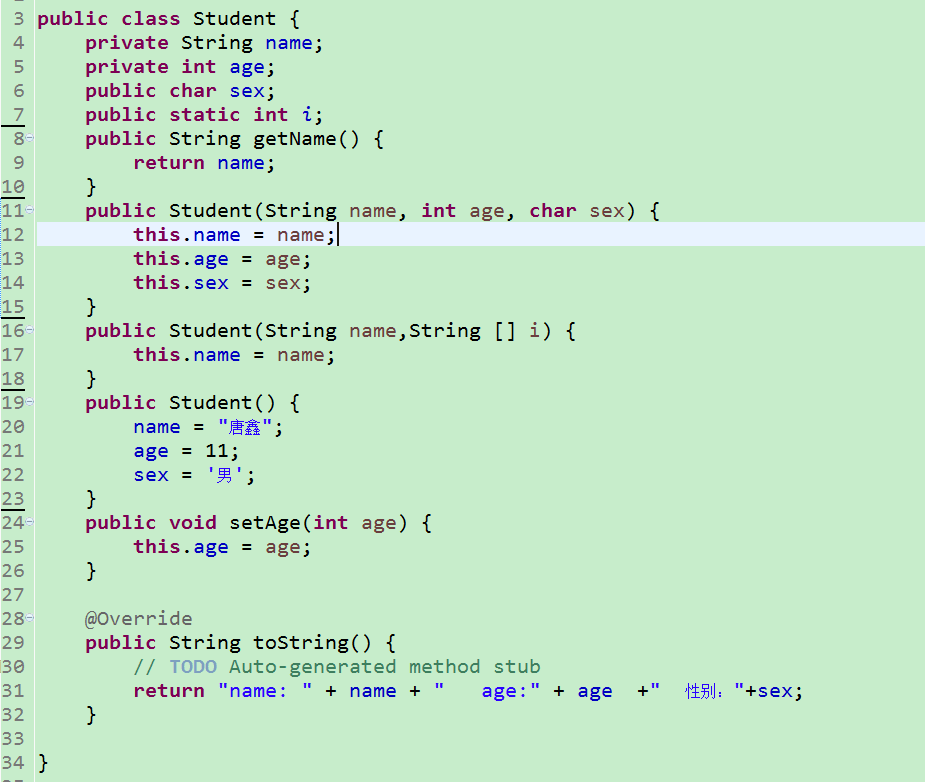
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101
| package com.share.eg01获取类的Class类的对象;
import java.lang.reflect.Constructor; import java.lang.reflect.Field; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method;
public class ClassTest { public static void main(String[] args) { getMethod(); } public static void getClassObject(){ Class<?> c1= Person.class; Person p=new Person(); Class<?> c2=p.getClass(); Class<?> c3=null; try { c3=Class.forName("com.share.eg01获取类的Class类的对象.Person"); } catch (ClassNotFoundException e) { e.printStackTrace(); } System.out.println(c1); System.out.println(c2); System.out.println(c3); } public static void getConstructor(){ Person p=new Person(); Class<?> c=p.getClass(); Constructor<?>[] constructors = c.getConstructors(); for(int i=0;i<constructors.length;i++){ System.out.println(constructors[i]); Class<?>[] pt = constructors[i].getParameterTypes(); for(int j=0;j<pt.length;j++){ System.out.println(pt[j]); } } System.out.println("----------------------"); for(Constructor co:constructors){ System.out.println(co); } } public static void getMethod(){ Person p=new Person(); Class<?> c=p.getClass(); Method[] methods = c.getDeclaredMethods(); for(int i=0;i<methods.length;i++){ System.out.println(methods[i].getName()); } try { methods[2].setAccessible(true); methods[2].invoke(p); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (IllegalArgumentException e) { e.printStackTrace(); } catch (InvocationTargetException e) { e.printStackTrace(); } } public static void getFeild(){ Person p=new Person(); Class<?> c=p.getClass(); Field[] fields = c.getDeclaredFields(); for(int i=0;i<fields.length;i++){ System.out.println(fields[i]); fields[i].setAccessible(true); } try { fields[0].set(p, "1"); System.out.println(p.getName()); } catch (IllegalArgumentException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| package com.share.eg01获取类的Class类的对象;
public class Person { private String name;
public Person() { super(); name="1"; }
public Person(String name) { super(); this.name = name; }
public String getName() { return name; }
public void setName(String name) { this.name = name; } public void show(){ System.out.println(name); } private void test(){ System.out.println(name); } }
|
实例2:
json数据解析
将通过URL获取服务器上的jason的数据并将数据进行解析
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13
| package com.share.eg02_JSON数据解析;
import com.google.gson.Gson;
public class JsonTest { public static void main(String[] args) { String s=GetJsonData.getJasonData(); Gson gson=new Gson(); Message m=gson.fromJson(s, Message.class); System.out.println("解析的结果:"+m); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.share.eg02_JSON数据解析;
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.net.MalformedURLException; import java.net.URL;
public class GetJsonData { public static String getJasonData(){ BufferedReader in=null; StringBuffer sb=new StringBuffer(); try { URL url=new URL("http://172.18.6.3:8080/PunishmentVote/vote?result=1"); InputStream is=url.openStream(); InputStreamReader isr=new InputStreamReader(is,"UTF-8"); in=new BufferedReader(isr); String inputLine; while((inputLine=in.readLine())!=null){ sb.append(inputLine); System.out.println(inputLine); } } catch (MalformedURLException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }finally{ try { in.close(); } catch (IOException e) { e.printStackTrace(); } } return sb.toString(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| package com.share.eg02_JSON数据解析;
public class Message { private String vote_result; private String result; public Message() { super(); } public Message(String vote_result, String result) { super(); this.vote_result = vote_result; this.result = result; }
public String getVote_result() { return vote_result; } public void setVote_result(String vote_result) { this.vote_result = vote_result; } public String getResult() { return result; } public void setResult(String result) { this.result = result; } @Override public String toString() { return "【vote_result】:"+vote_result+" 【result】:"+result; } }
|
实例3:
json数据解析
解析下面的数据:
{
“resultcode”: “200”,
“reason”: “successed!”,
“result”: {
“sk”: {
“temp”: “36”,
“wind_direction”: “西风”,
“wind_strength”: “2级”,
“humidity”: “50%”,
“time”: “11:23”
},
“today”: {
“temperature”: “30℃38℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “南风微风”,
“week”: “星期六”,
“city”: “苏州”,
“date_y”: “2016年07月23日”,
“dressing_index”: “炎热”,
“dressing_advice”: “天气炎热,建议着短衫、短裙、短裤、薄型T恤衫等清凉夏季服装。”,
“uv_index”: “很强”,
“comfort_index”: “”,
“wash_index”: “较适宜”,
“travel_index”: “较适宜”,
“exercise_index”: “较适宜”,
“drying_index”: “”
},
“future”: [
{
“temperature”: “30℃38℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “南风微风”,
“week”: “星期六”,
“date”: “20160723”
},
{
“temperature”: “30℃38℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “东南风微风”,
“week”: “星期日”,
“date”: “20160724”
},
{
“temperature”: “30℃38℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “东南风微风”,
“week”: “星期一”,
“date”: “20160725”
},
{
“temperature”: “30℃39℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “西南风微风”,
“week”: “星期二”,
“date”: “20160726”
},
{
“temperature”: “30℃38℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “西风微风”,
“week”: “星期三”,
“date”: “20160727”
},
{
“temperature”: “30℃39℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “西南风微风”,
“week”: “星期四”,
“date”: “20160728”
},
{
“temperature”: “30℃38℃”,
“weather”: “晴”,
“weather_id”: {
“fa”: “00”,
“fb”: “00”
},
“wind”: “东南风微风”,
“week”: “星期五”,
“date”: “20160729”
}
]
},
“error_code”: 0
}
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| package com.share.eg03_Json数据解析;
public class Future { private String temperature; private String weather; private Weather_id wheather_id; private String wind; private String week; private String date; public Future() { super(); } public Future(String temperature, String weather, Weather_id wheather_id, String wind, String week, String date) { super(); this.temperature = temperature; this.weather = weather; this.wheather_id = wheather_id; this.wind = wind; this.week = week; this.date = date; } public String getTemperature() { return temperature; } public void setTemperature(String temperature) { this.temperature = temperature; } public String getWeather() { return weather; } public void setWeather(String weather) { this.weather = weather; } public Weather_id getWheather_id() { return wheather_id; } public void setWheather_id(Weather_id wheather_id) { this.wheather_id = wheather_id; } public String getWind() { return wind; } public void setWind(String wind) { this.wind = wind; } public String getWeek() { return week; } public void setWeek(String week) { this.week = week; } public String getDate() { return date; } public void setDate(String date) { this.date = date; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| package com.share.eg03_Json数据解析;
public class JsonData { private int resultcode; private String reason; private Result result; private int error_code; public JsonData() { super(); } public JsonData(int resultcode, String reason, Result result, int error_code) { super(); this.resultcode = resultcode; this.reason = reason; this.result = result; this.error_code = error_code; } public int getResultcode() { return resultcode; } public void setResultcode(int resultcode) { this.resultcode = resultcode; } public String getReason() { return reason; } public void setReason(String reason) { this.reason = reason; } public Result getResult() { return result; } public void setResult(Result result) { this.result = result; } public int getError_code() { return error_code; } public void setError_code(int error_code) { this.error_code = error_code; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| package com.share.eg03_Json数据解析;
import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method;
import com.google.gson.Gson;
public class JsonTest { public static void main(String[] args) { String jsonString = "{\"resultcode\":\"200\",\"reason\":\"successed!\",\"result\":{\"sk\":{\"temp\":\"36\",\"wind_direction\":\"西风\",\"wind_strength\":\"2级\",\"humidity\":\"50%\",\"time\":\"11:23\"},\"today\":{\"temperature\":\"30℃~38℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"南风微风\",\"week\":\"星期六\",\"city\":\"苏州\",\"date_y\":\"2016年07月23日\",\"dressing_index\":\"炎热\",\"dressing_advice\":\"天气炎热,建议着短衫、短裙、短裤、薄型T恤衫等清凉夏季服装。\",\"uv_index\":\"很强\",\"comfort_index\":\"\",\"wash_index\":\"较适宜\",\"travel_index\":\"较适宜\",\"exercise_index\":\"较适宜\",\"drying_index\":\"\"},\"future\":[{\"temperature\":\"30℃~38℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"南风微风\",\"week\":\"星期六\",\"date\":\"20160723\"},{\"temperature\":\"30℃~38℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"东南风微风\",\"week\":\"星期日\",\"date\":\"20160724\"},{\"temperature\":\"30℃~38℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"东南风微风\",\"week\":\"星期一\",\"date\":\"20160725\"},{\"temperature\":\"30℃~39℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"西南风微风\",\"week\":\"星期二\",\"date\":\"20160726\"},{\"temperature\":\"30℃~38℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"西风微风\",\"week\":\"星期三\",\"date\":\"20160727\"},{\"temperature\":\"30℃~39℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"西南风微风\",\"week\":\"星期四\",\"date\":\"20160728\"},{\"temperature\":\"30℃~38℃\",\"weather\":\"晴\",\"weather_id\":{\"fa\":\"00\",\"fb\":\"00\"},\"wind\":\"东南风微风\",\"week\":\"星期五\",\"date\":\"20160729\"}]},\"error_code\":0}"; System.out.println(jsonString); JsonData data = new Gson().fromJson(jsonString, JsonData.class);
Today today = data.getResult().getToday(); Method[] methods = today.getClass().getDeclaredMethods();
try { for (int i = 0; i < methods.length; i++) { if (methods[i].getName().equals("getWeather_id")) { System.out.println(methods[i].getName().substring(3).toLowerCase() + "="); Weather_id weathre_id = (Weather_id) methods[i].invoke(today); Method[] methods2 = weathre_id.getClass().getDeclaredMethods(); for (Method m : methods2) { if (m.getName().startsWith("get")) { System.out.println( "\t" + m.getName().substring(3).toLowerCase() + "=" + m.invoke(weathre_id)); } } continue; } if (methods[i].getName().startsWith("get")) { System.out .println(methods[i].getName().substring(3).toLowerCase() + "=" + methods[i].invoke(today)); } } } catch (IllegalAccessException e) { e.printStackTrace(); } catch (IllegalArgumentException e) { e.printStackTrace(); } catch (InvocationTargetException e) { e.printStackTrace(); } } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| package com.share.eg03_Json数据解析;
import java.util.List;
public class Result { private SK sk; private Today today; private List<Future> future; public Result() { super(); } public Result(SK sk, Today today, List<Future> future) { super(); this.sk = sk; this.today = today; this.future = future; } public SK getSk() { return sk; } public void setSk(SK sk) { this.sk = sk; } public Today getToday() { return today; } public void setToday(Today today) { this.today = today; } public List<Future> getFuture() { return future; } public void setFuture(List<Future> future) { this.future = future; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| package com.share.eg03_Json数据解析;
public class SK { private String temp; private String wind_direction; private String wind_strength; private String humidity; private String time; public SK() { super(); } public SK(String temp, String wind_direction, String wind_strength, String humidity, String time) { super(); this.temp = temp; this.wind_direction = wind_direction; this.wind_strength = wind_strength; this.humidity = humidity; this.time = time; } public String getTemp() { return temp; } public void setTemp(String temp) { this.temp = temp; } public String getWind_direction() { return wind_direction; } public void setWind_direction(String wind_direction) { this.wind_direction = wind_direction; } public String getWind_strength() { return wind_strength; } public void setWind_strength(String wind_strength) { this.wind_strength = wind_strength; } public String getHumidity() { return humidity; } public void setHumidity(String humidity) { this.humidity = humidity; } public String getTime() { return time; } public void setTime(String time) { this.time = time; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135
| package com.share.eg03_Json数据解析;
public class Today { private String temperature; private String weather; private Weather_id weather_id; private String wind; private String week; private String city; private String date_y; private String dressing_index; private String dressing_advice; private String uv_index; private String comfort_index; private String wash_index; private String travel_index; private String exercise_index; private String drying_index; public Today() { super(); } public Today(String temperature, String weather, Weather_id weather_id, String wind, String week, String city, String date_y, String dressing_index, String dressing_advice, String uv_index, String comfort_index, String wash_index, String travel_index, String exercise_index, String drying_index) { super(); this.temperature = temperature; this.weather = weather; this.weather_id = weather_id; this.wind = wind; this.week = week; this.city = city; this.date_y = date_y; this.dressing_index = dressing_index; this.dressing_advice = dressing_advice; this.uv_index = uv_index; this.comfort_index = comfort_index; this.wash_index = wash_index; this.travel_index = travel_index; this.exercise_index = exercise_index; this.drying_index = drying_index; } public String getTemperature() { return temperature; } public void setTemperature(String temperature) { this.temperature = temperature; } public String getWeather() { return weather; } public void setWeather(String weather) { this.weather = weather; } public Weather_id getWeather_id() { return weather_id; } public void setWeather_id(Weather_id weather_id) { this.weather_id = weather_id; } public String getWind() { return wind; } public void setWind(String wind) { this.wind = wind; } public String getWeek() { return week; } public void setWeek(String week) { this.week = week; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } public String getDate_y() { return date_y; } public void setDate_y(String date_y) { this.date_y = date_y; } public String getDressing_index() { return dressing_index; } public void setDressing_index(String dressing_index) { this.dressing_index = dressing_index; } public String getDressing_advice() { return dressing_advice; } public void setDressing_advice(String dressing_advice) { this.dressing_advice = dressing_advice; } public String getUv_index() { return uv_index; } public void setUv_index(String uv_index) { this.uv_index = uv_index; } public String getComfort_index() { return comfort_index; } public void setComfort_index(String comfort_index) { this.comfort_index = comfort_index; } public String getWash_index() { return wash_index; } public void setWash_index(String wash_index) { this.wash_index = wash_index; } public String getTravel_index() { return travel_index; } public void setTravel_index(String travel_index) { this.travel_index = travel_index; } public String getExercise_index() { return exercise_index; } public void setExercise_index(String exercise_index) { this.exercise_index = exercise_index; } public String getDrying_index() { return drying_index; } public void setDrying_index(String drying_index) { this.drying_index = drying_index; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package com.share.eg03_Json数据解析;
public class Weather_id { private String fa; private String fb; public Weather_id() { super(); } public Weather_id(String fa, String fb) { super(); this.fa = fa; this.fb = fb; } public String getFa() { return fa; } public void setFa(String fa) { this.fa = fa; } public String getFb() { return fb; } public void setFb(String fb) { this.fb = fb; } }
|