2017-04-19-javaScript(DOM)
知识点1:
学习方法论:
实例1:
遍历一个HTML网页的DOM文档对象模型的结构树
【多练几遍】
思路:
通过递归来遍历结构树,通过全局变量来存储遍历的节点的名称,并将节点的个数通过返回值返回到调用处,最后将节点名称和节点个数通过警告弹窗将信息展示出来。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| <!DOCTYPE html>
<html> <head> <title>js获取DOM</title> </head> <body onload="show()"> 遍历HTML网页中的所有元素节点</br> <a href="https://www.baidu.com">百度</a> </body> <script type="text/javascript"> var elementList=""; function getElement(node){ var total=0; if(node.nodeType==1){ total++; elementList=elementList+node.nodeName+","; } var childrens=node.childNodes; for(var m=node.firstChild;m!=null;m=m.nextSibling){ total+=getElement(m); } return total; } function show(){ var number=getElement(document); elementList=elementList.substring(0,elementList.length-1); alert("该文档中包含:"+elementList+"等"+number+"个标记!"); elementList=""; } </script> </html>
|
实例2:
动态生成和删除有序列表的元素
【多练几遍】
思路:
通过document获取标签对象来进行操作
添加直接添加就行了
删除则直接删除最后一个就行了,
为了防止删除最后的两个元素怎有三种思路:
1.设置大于5
2.设置标志
3.设置id
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| <!DOCTYPE html>
<html> <head> <title></title> </head> <body> <div> <ol id="orderList"> <li>张三</li> <li>李四</li> </ol> </div> <div> <input type="text" id="text" /> <input type="button" id="bt" onclick="add()" value="添加" /> <input type="button" id="bt1" onclick="rem()" value="删除" /> </div> </body> <script type="text/javascript"> var list=document.getElementById("orderList"); var text=document.getElementById("text"); var bt=document.getElementById("bt"); var i=0; function add(){ i=i+1; var child=document.createElement("li"); var txt=document.createTextNode(text.value); child.appendChild(txt); list.appendChild(child); text.value=""; } function dele(){ var child=list.lastChild; list.removeChild(child); } function remo(){ var children=list.childNodes; var childNum=children.length; var child=list.lastChild; if(childNum>5){ list.removeChild(child); } } function rem(){ if(i!=0){ var child=list.lastChild; list.removeChild(child); i-=1; } } </script> </html>
|
注意:在button中添加一个remove()方法,不论有没有这个自定义方法,会直接将这个button直接删除掉,还有clear()方法是js中的固定方法,清楚一般写为clean()。
特殊知识点:

ol的子节点其实有5个,是因为即使文本1,2,3都没有写,仍然有空白的文本存在,
这证明了一句话:“有些东西看不见,并不代表它不存在。”
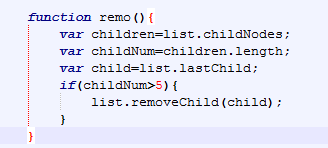
实例4:
js中实现霓虹灯效果
【多练几遍】
思路:
将色码表中的几个数字存进数组中,在js中设置定时器调用方法调用一次后取出数组中的一个颜色,然后计数标志加1,当计数标志等于数组下标的最大值的时候就开始重新设置。实现有序显示颜色。
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <!DOCTYPE html>
<html> <head> <title></title> </head> <body onload="change()"> <table id="table1" border=10> <tr> <td>11111111</td> </tr> <tr> <td>22222222222222</td> </tr> </table> </body> <script type="text/javascript"> var i=0; var colors=new Array("#0000FF","#99FF00","#660033","#cc66cc","#FFFF33"); function change(){ if(i>colors.length-1){ i=0; } table1.style.borderColor=colors[i]; i+=1; setTimeout("change()",500); } </script> </html>
|
实例5:
js实现论坛的评价的添加和删除的效果
思路:
添加删除元素
核心代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124
| <!DOCTYPE html>
<html> <head> <title>论坛</title> <style> textarea { width:334px; height:100px; } td { background-color:#77FF00; } .re { background-color:#cccccc; } #reviewer { width:336px; height:20px; } #td1 { width:100px; height:20px; background-color:orange; } #td2 { width:320px; height:20px; background-color:orange; } #table1 { background-color:yellow; } #div2 { width:431px; height:200px; background-color:gray; } </style> </head> <body> <center> <div> <img src="socialNetwork.png"> </div> <div> <table border="0" id="table1"> <tr> <td id="td1">评论人</td> <td id="td2">评论内容</td> </tr> </table> </div> <p></p> <div id="div2"> <form> <table> <tr> <td class="re">评论人:</td> <td class="re"><input type="text" id="reviewer"/></td> </tr> <tr> <td class="re">评论内容:</td> <td class="re"><textarea rows="" cols="" id="review"></textarea></td> </tr> <tr> <td class="re"></td> <td class="re"> <input type="button" onclick="add()" value="发表"> <input type="reset" onclick="" value="重置"> <input type="button" onclick="deleteFirst()" value="删除第一条评论"> <input type="button" onclick="deleteLast()" value="删除最后一条评论"> </td> </tr> </table> </form> </div> </center> </body> <script type="text/javascript"> var reviewer=document.getElementById("reviewer"); var review=document.getElementById("review"); var table1=document.getElementById("table1"); var flag=0; function add(){ flag=flag+1; var tr1=document.createElement("tr"); var td1=document.createElement("td"); var td2=document.createElement("td"); var txt1=document.createTextNode(reviewer.value); var txt2=document.createTextNode(review.value); td1.appendChild(txt1); td2.appendChild(txt2); tr1.appendChild(td1); tr1.appendChild(td2); table1.appendChild(tr1); reviewer.value=""; review.value=""; } function deleteFirst(){ if(flag!=0){ var children=table1.childNodes; table1.removeChild(children[2]); flag-=1; } } function deleteLast(){ if(flag!=0){ var child=table1.lastChild; table1.removeChild(child); flag-=1; } } </script> </html>
|